Recent Posts
Rambles in Machine Learning
21. February, 2019
Classifying Chatrooms with scikit-learn
Machine Learning has been ascending in the Zeitgest for long enough that I'm probably behind the curve, but that wasn't going to prevent me from having a shoofty (apparently the most common spelling is "shufti" but never mind). Since python has been my programming home since at least 2009, scikit-learn was the obvious choice of tool. (unfortunately my projects folder's metadata got nuked during a file-transfer in 2009. Looking through now, my earliest verifiable python project was pyGenes, a Creatures 3 genome editor, which existed by 2005!)
Last year I bought Introduction to Machine Learning with Python along with a bunch of other Machine Learning books as part of a humble bundle, and had already read through it (though without actually writing any code myself... an activity which may be of questionable value) so I had a reasonable idea of what kind of toolkit it was. Having had the idea to attempt some ML project back then the key question was of course what I could investigate.
I didn't want to just play with the included data --- something done to death and quite boring, no matter how cool training handwriting classifiers on MNIST is (and anyway, you do this in coursera's Machine Learning course). But there are very few datasets that one already has that are suitable for the task, especially when one considers that I am far too lazy to hand-label more than about ten lines of data before getting bored and giving up. Unsupervised learning is always a possibility, but it lacks easily-quantifed metrics of success and there is the very real risk that you need genuine insight and intelligence to do something interesting. I thought about collecting League of Legends data and deriving stats and strategies from that... but many people are doing this already, probably much better than me and then I finally followed the advice of all my teammates over the years and uninstalled the game, so I also lost the personal interest.
Thankfully, a trusty piece of technology invented in the year of my birth came to the rescue: IRC. Thanks to Quassel I have chatroom logs dating back to 2012: millions of lines from thousands of people, all ready-labeled with all sorts of metadata and in a convenient Postgres database. Even my discord chats are routed through Quassel using bitlbee (I refuse to come back to Slack now they killed off the IRC transport) My first idea: predicting from lines of chat which chatroom it was said in. This already offers a bunch of routes of exploration in terms of pre-processing, model selection and tuning, and is just the kind of supervised Natural Language Processing classification task that people have been doing for ages.
In fact the scikit-learn documentation has a tutorial on text data which covers exactly this kind of scenario, only classifying BBS posts into their newsgroups instead of IRC messages into channels. Before I discovered this I had been fiddling around with textacy, but the key feature I needed from textacy for the initial experiments was a vectorizer (transforming text into a sparse array where a[i] = n
means that there were occurrences of the word with index
in some enumeration of words) and it turns out scikit-learn itself contains some options for this.
So for this initial project I set the following parameters:
-
Attempt to classify which of five IRC/Discord channels lines of text came from
-
Use scikit-learn's
SGDClassifier
(i.e. an SVM) since that's what the tutorial does -
Use a grid-search to find some decent parameters
-
Remove nicknames from the text
The last one was something I decided I wanted to do early on --- if IRCUser42 is only present in #channel1, then any line that happens to be directly addressed to her (or mentions her name) will of course be easy to classify. We want to set the model a challenge!
Here's the code I used to train the model and print some statistics about it:
import numpy as np from sklearn.feature_extraction.text import CountVectorizer from sklearn.model_selection import train_test_split, GridSearchCV from sklearn.linear_model import SGDClassifier from sklearn.preprocessing import StandardScaler from sklearn.pipeline import Pipeline from sklearn import metrics def train(data, labels, nicks): vectorizer = CountVectorizer(ngram_range=(1,3)) tokenize = vectorizer.build_tokenizer() preprocess = vectorizer.build_preprocessor() stop_words = set() for n in nicks: stop_words |= set(tokenize(preprocess(n))) vectorizer.stop_words = stop_words tfidf = sklearn.feature_extraction.text.TfidfTransformer() scaler = StandardScaler(with_mean=False) classifier = SGDClassifier(penalty='l1', alpha=0.002, random_state=43, max_iter=5) pipeline = Pipeline([ ('vect', vectorizer), ('tfidf', tfidf), ('scaler', scaler), ('clf', classifier)]) parameters = { 'vect__ngram_range': [(1,1),(1,2),(1,3)], 'tfidf__use_idf': (True, False), 'clf__alpha': (1e-1, 1e-2, 1e-3), 'clf__penalty': ('l1', 'l2') } #if cv is low, best_score_ will be low, but the training/test scores may still be high. search = GridSearchCV(pipeline, parameters, cv=3, iid=True, n_jobs=-1) X_train, X_test, y_train, y_test = train_test_split(data, labels, random_state=43, stratify=labels) #model.fit(X_train, list(y_train)) model = search.fit(X_train, y_train) print('Best parameters:', model.best_params_) print('Best CV score: %.2f' % (model.best_score_)) print('Training score: %.2f' % (model.score(X_train, y_train))) print('Test score: %.2f' % (model.score(X_test, y_test))) predicted = model.predict(X_test) print(metrics.classification_report(y_test, predicted)) print(metrics.confusion_matrix(y_test, predicted)) return model
```
data
and labels
should be the lines of chat and channel names, which can be obtained from Quassel's backlog database, as numpy arrays. nicks
should be a list of nicknames to filter from the text. We just use the Vectorizer's stop_words
attribute for this so we could use this to filter out anything. It's worth pointing out that many nicknames are real English words. It would be crazy to filter those out, so when generating the list of nicks, we throw out anything in an English vocabulary list. This is the only bit of the process which is language-dependent.
I run the model like this:
>>> train(rows[:,0], rows[:,1], nicks) Best parameters: {'tfidf__use_idf': True, 'clf__alpha': 0.01, 'clf__penalty': 'l2', 'vect__ngram_range': (1, 1)} Best CV score: 0.54 Training score: 0.76 Test score: 0.56 precision recall f1-score support #Ins.general-chat 0.57 0.47 0.51 1000 #Str.general-chat 0.56 0.45 0.50 1000 #latex 0.64 0.70 0.67 1000 #notpron 0.46 0.56 0.51 1000 #quassel 0.57 0.61 0.59 1000 micro avg 0.56 0.56 0.56 5000 macro avg 0.56 0.56 0.56 5000 weighted avg 0.56 0.56 0.56 5000 [[470 128 76 207 119] [164 449 69 212 106] [ 39 45 705 102 109] [101 120 102 556 121] [ 56 60 151 119 614]]
A null model which just guessed one class all the time would score 20% so this isn't too bad, but it isn't great, either. It's worth noting that since the classes are (almost) equal in size, the accuracy score is a reasonable summary metric. It's no more important to correctly classify lines from #latex as coming from #latex than it is to, say, avoid incorrectly labelling lines as coming from #quassel. I was surprised that the grid search found that ngrams were worse than bags of words for accuracy. This means that the model was best able to learn purely from the vocabulary used in the different channels, and not from the patterns of words at all.
Let's look at the confusion matrix visually:
import matplotlib.pyplot as plt def plot_confusion_matrix(cm, classes): cm_norm = cm.astype('float')/cm.sum(axis=1)[:, np.newaxis] cmap = plt.cm.get_cmap('Blues') plt.imshow(cm, interpolation='nearest', cmap=cmap) plt.colorbar() plt.title('Confusion Matrix of SVM') plt.xlabel('Prediction') plt.ylabel('Channel') tick_marks=np.arange(len(classes)) plt.xticks(tick_marks, classes, rotation=20, ha='right') plt.yticks(tick_marks, classes) import itertools for i, j in itertools.product(range(cm.shape[0]), range(cm.shape[1])): plt.text(j, i, cm[i, j], ha='center', color='white' if cm_norm[i,j] > 0.5 else 'black') plt.tight_layout()
Running this gives us:
From this we can easily see where the model did badly and where it did better. #latex and #quassel were the easiest to classify — no surprise since they are technical support channels with the attendant unique jargon. The other three channels are general chat channels and harder to distinguish. #Ins.general-chat and #Str.general-chat in particular are both video game focused with overlapping user bases, so the fact they were the hardest to classify is also not surprising, but it's worth pointing out that they were both mis-labeled more often as #notpron than as each other, which is odd. While the two general-chats were less often mis-labeled as #latex, #quassel was more often, presumably because of general tech support vocab.
How can we improve the accuracy? An easy way would be to make the problem easier! Something you don't always have the luxury of doing but while playing around, why not. Reducing the number of classes makes things a lot easier — classifying between two channels got over 70% accuracy with no tuning. But this is pretty boring. On the other hand it's not unreasonable to feed the model more data at a time by concatenating several lines of chat. We can do this with the same data from the database as before, contained in rows
:
def chunks(l, n): for i in range(0, len(l), n): yield l[i:i + n] joined = [] joined_label = [] for rr, l in zip(chunks(rows[:,0], 4), rows[:,1][::4]): joined.append('\n'.join(rr)) joined_label.append(l)
Rerunning train
gives us a test score of 63%, a marked improvement — especially since we didn't actually give the model any more data, just fed it more at once. If we instead retrieve more rows from the database to jam together, say 25,000 from each channel and stick together 5 at once, we get the following parameters and scores:
Best parameters: {'tfidf__use_idf': True, 'clf__alpha': 0.001, 'clf__penalty': 'l2', 'vect__ngram_range': (1, 1)} Best CV score: 0.74 Training score: 0.86 Test score: 0.76 precision recall f1-score support #Ins.general-chat 0.80 0.53 0.64 753 #Str.general-chat 0.77 0.70 0.73 1247 #latex 0.76 0.95 0.84 1250 #notpron 0.74 0.65 0.69 1250 #quassel 0.75 0.88 0.81 1250 micro avg 0.76 0.76 0.76 5750 macro avg 0.76 0.74 0.74 5750 weighted avg 0.76 0.76 0.75 5750 [[ 399 133 47 102 72] [ 62 875 57 143 110] [ 1 7 1182 12 48] [ 30 107 164 810 139] [ 6 17 100 24 1103]]
Oops, it looks like there weren't that many qualifying lines from the first two channels, though overall we are doing much better. A null model here would still only get an accuracy of 22%. You can see that the model hardly misclassifies anything as #Ins.general-chat (best precision) but misclassifies it as other things more frequently than any other channel. Nevertheless the recall is slightly improved compared to the situation with equal classes and less data. Let's visualise the confusion matrix again (normalised to the support):
The cross-validation is still finding that ngrams don't help. This does mean that we can easily examine what are the most important words for classification. The SVM stores its coefficients under the coef_
parameter and we can get an idea of which words the model thinks are associated with being in - or not being in - each category by whether the corresponding coefficient is strongly positive or strongly negative. We use numpy.argsort
to return the indices of the largest coefficients, and retrieve the associated word from the vectoriser with get_feature_names()[index]
.
# Retrieve the vectoriser and SVM portions of the pipeline vec = model.best_estimator_.steps[0][1] svm = model.best_estimator_.steps[3][1] # Retrieve the relation from vector --> word rev = vec.get_feature_names() for i, c in enumerate(model.classes_): sig = np.argsort(svm.coef_[i,:])[-25:] words = [rev[n] for n in sig] print(c) print(" " + " ".join(words))
#Ins.general-chat: picker hyped beans minutes i65 seat expo 26th 289695103902285824 clan 30 i64 tickets loyalty 2019 remaining cooldown 64 byoc months mere 18th hello 22nd insomnia #Str.general-chat: blur roast liab grandstand warranty paddock car fileserver attachments prop heating 448872115081314314 announcement dinner pudding pinned boiler tomorrow stratlan racecourse supcom kitchen ayce golf 266234897633509376 #latex: inside math environments file stackexchange page xetex section pdflatex xelatex pdf org begin paste pastebin environment texlive sample aborts package ctan compiles align document latex #notpron: face riddle kinder silly wind trivia song coverage doctor humidity bgsound blowing mbar apartment country egg weather views university lounge fish finland trump url2title swedish #quassel: notification version bugs windows saint connecting connection channels database messages postgresql notifications quasselsuche build builds user backlog connect quasselcore sqlite quasseldroid clients protocol client core
A couple of things are obvious from this analysis: firstly, the long numbers. Some of these come from bitlbee (failing to) render discord roles so that "@Staff" turns into a long identification number for the "Staff" role. Others come from common URL components that discord re-hosts images under. It's tricky to know the best way to deal with these - on the one hand it seems to be cheating to use some computer-generated unique identifiers. On the other, filtering out URLs - or numbers - entirely seems over the top.
"url2title" under #notpron is a label that a bot in the channel uses when it automatically prints the titles of webpages participants link. This is therefore not representative of the human participation in the channel, and an enhancement could involve filtering out bots' messages. In that channel users can also type "!weather <location>" (the source of "weather" in the list) to receive weather information from the bot, which is the source of many words there. Some words in other channels also come from bots and bot triggers.
I chose not to lemmatise the words before training because I thought that different grammar patterns in different channels might help distinguish them. In a few cases this does seem to be the case, but mostly not. Here is an example where it makes a difference:
>>> svm.coef_[:,vec.vocabulary_.get('train')] array([ 0.00082995, -0.00049029, -0.00435588, 0.00564122, -0.00626548]) >>> svm.coef_[:,vec.vocabulary_.get('training')] array([-0.00259072, 0.00241011, -0.00315301, 0.00380725, -0.00373427])
We see that the importance of the word flips in two cases, as well as changing a bit in others. The effect here is stronger than in most cases I checked, presumably because "training" is a completely different meaning from "a train." Rather than picking up different grammar, not lemmatising allows us to detect different meanings of words that might be squashed otherwise.
One last comment is that the #latex list is full of non-English jargon like "xetex" and "texlive," and even the real English words will be familiar to LaTeX users as being keywords in the language. Real language is littered with words that aren't in the dictionary, whether typos, slang or local jargon and it's not really possible to clean this up without removing the essential character of the chat.
All these factors affect my perception of the "fairness" of the exercise - a vague sense that when setting yourself a learning exercise you shouldn't make it too easy. Still, they are difficult to mitigate and don't really defeat the purpose.
i61 iHunt: Guesses Hall of Fame
2. September, 2017
"CAN THE IHUNT TEAM STILL SEE EVERYTHING ENTERED???" — Steampunk_Sam (RGBeeGees) for From the Shadows
Yes, yes we can. And so we thought we'd bring you some of our favourite guesses from the iHunt at i61.
In total, there were 9635 guesses, (of which 77 were trying the transmission content from the finale!) The person who guessed the most was Manituo with 485 guesses, and the team which guessed the most was The Pieces of Shmitt with 850 guesses - representing 5% and 9% of the total guesses each! JGBrock178, who came fourth on a solo team, made more guesses on his own than the entire team of Saor Gamers, who came second.
"sir lampy mclampface" — Tarrenam (XW) for Hunt the Sailors
I'm not sure which lamp that referred to.
"The community team area is about 95 miles away. :(" — Dataforce for Hunt the Sailors
That's your fault for not coming to the LAN >:(
"cock semaphore" — Manituo for Hunt the Sailors
"penis semaphore" — Manituo for Hunt the Sailors
Our reply: "Well HELLO, sailor! Your flagpole is looking very... shiny!"
"64 gallons of Napalm" — Taitenator (Crippling Depression) for From the Shadows
"The Light of Elendil" — Taitenator (Crippling Depression) for From the Shadows
That would indeed light things up!
"Theihuntteamareallevilandneedtobeducttapedtoeachotheronstage" — ZeroG for Mixed Messages
Some of the iHunt team would pay for that privilege.
In response to "you must carefully choose which portal you want to open":
"The one with nice things behind it please" — sedders123 (Crippling Depression) for Mixed Messages
People failing at Mario:
"why didn't they just give mario a gun" — jith100 for Let's-a go!
"wait what the fuck you can shoot oh my god" — jith100 for Let's-a go!
"cba m8 just give me the answer ty" — jith100 for Let's-a go!
"i keep fucking dying " — Manituo for Let's-a go!
"fucking hell I am inept at this game" — jith100 for Let's-a go!
"blow it out your ass" — BraveSerRobin (Two And A Half Programmers) for Where am I?
Our reply: "I'M GONNA RIP OFF YOUR HEAD AND SHIT DOWN YOUR NECK"
"if you're not careful I'm going to upload a bunch of really grim pictures" — jith100 for Open your Windows
Bring it on, jith :3
"that may have been my icloud password... lol" — chuff (Yellows_r_us) for A-maze-ing
Chuffing it up, every LAN! Tell me, how many lights do you see?
"there are four lights" — chuff (Yellows_r_us) for Burn After Writing
"Turn it off and on again" — sedders123 (Crippling Depression) for Burn After Writing
"this isn't even a qr code" — jith100 for Burn After Writing
Correct, Jith.
"gary motherfucking oak" — iagowynne (Null reference pointer) for A Pixelated but Impressively-Executed Texture
"Give me afucking HINT DAMN IOT" — Rossiboi (The Mighty Mongoloids) for A Pixelated but Impressively-Executed Texture
Your frustration sustain us :) Having difficulty spelling:
"Ultra High Defintion" — Manituo for A Pixelated but Impressively-Executed Texture
"ULTRA HIGH DEFINTION" — Manituo for A Pixelated but Impressively-Executed Texture
"ultra high definiton" — Manituo for A Pixelated but Impressively-Executed Texture
I had no idea this guy existed but what an awesome name:
"Piet Pieterszoon Hein" — Leithall (TaylorMoon) for A Pixelated but Impressively-Executed Texture
Issues with our amazing quadruple-A game:
"Out of habit, I keep trying to crouch and run forward. (Ctrl+W)..." — Dataforce for Safe and Sound
"this game is a 6.2 out of 10 too many tables" — BraveSerRobin (Two And A Half Programmers) for Safe and Sound
"The really terrible chair textures are amusing" — Dataforce for Safe and Sound
"I don't even know why I'm still running around this, as I can't go "here"" — Dataforce for Safe and Sound
"0118 999 88199 9119 725 ... 3" — Steampunk_Sam (RGBeeGees) for Safe and Sound
Two people on the iHunt team didn't recognise this number and thought you were just guessing random digits. They have been sent for re-education.
"tnetennba" — jith100 for A Garman
"tnetennba" — chuff (Yellows_r_us) for A Garman
"as if tnetennba isn't an unlock" — jith100 for A Garman
You make a good point. Unfortunately the person responsible is already in re-education. (We also added one just for you)
"I like cheese" — ZeroG for A Garman
That's nice ZeroG. Our reply: "We'll come round and blast out YMCA at you"
"I don't want to disturb the guy sitting there" — CodIsAFish (The A Team) for Through the Tubes
That's not how iHunt works!
"thebiggestblackestdick" — Danderlyon (Victorious Secret) for Through the Tubes
Did you mean to put that in pornhub?
Some people got a bit frustrated and typed a lot of garbage:
"qwetqwtgasdg]asd;'/fg]sadg];asd]g;as]dg;]dsagasdg]asdrfasdsadfgasdfr]#" — Rossiboi (The Mighty Mongoloids) for Interchange your Information
"agagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagagggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaggggggggggggggggggggggggggggggaaaaaaaaaaaaaaaaaaaaaaaaaaaagggggggggggggggggggggggggaaaaaaaaaaaaaaaaaaagggggggggggggggaaaaaaaaaaaaaaaaaaaggggggggggggggggggggggggggaaaaaaaaaaaaaaaaaaaaaagggggggggggggggggggggggggggggggggggaaaaaaaaaaaaaaaaaaaaagggggggggggggggggggggggggggggggaaaaaaaaaaaaaaaaaaaaggggggggggggaaaaaaaaaaaaggggggggggggggaaaaaaaaaaaaagggggggggggggaaaaaaaaaaaaagggggggggggggaaaaaaaaaaaaggggggggggggggggagagagagagagagagagagagagagagagagagagagagagagaggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggggaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaagaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaagaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaagaaaaaaaaaaaaaaaaaaaaaaaaaa" — ZeroG for Mixed Messages
"MTk2MzExMjExNzExNDEwMzEwMTAwMA==" — Takamaru (Bruce Hindsyth) for Interchange your Information
We actually decoded some of your base64, I hope you're happy. People had trouble deciphering my layered text without the super-secret method to separate the layers:
"he washed but he had eaten 72 cats" — Leithall (TaylorMoon) for Micro- and Macroscopics
"areola" — jith100 for Micro- and Macroscopics
Our reply: "we're rubbing ours"
"0118 999 88199 9119 725" — Deathmendockx (RGBeeGees) for Stepping Stones
You missed the 3!
"this one makes me sad... enjoy my tears!" — netvyper (Step on snek) for Stepping Stones
"walking through this is almost as arduous as actually walking through the NEC" — jith100 for Stepping Stones
Goooood.
"He protec but he also attac" — sedders123 (Crippling Depression) for The Trailblazer
Our reply: "Who? Do you mean Conan? He only attac. Need healing :("
"tank and spank" — Niyxar for The Trailblazer
Our reply: "Meet me at M19 bb"
"but most importantly" — CodIsAFish (The A Team) for The Trailblazer
"What the fuck is this" — CodIsAFish (The A Team) for The Trailblazer
"helpful hints as ever guys :D" — Xik for The Trailblazer
:3
"the three faces of fear" — Rossiboi (The Mighty Mongoloids) for Rogueish
"MAKE IT MORE RETRO THEN" — Danderlyon (Victorious Secret) for Rogueish
"Wally the Wonder Badger" — Lavinder (m0nkeynutz) for Rogueish
I want to meet this badger.
"Dominos garlic and herb" — CSaur (Invaders) for Watch Your Step
"are you sure its not garlic and herb" — CSaur (Invaders) for Watch Your Step
I have no idea who put that dip at the end of that trail, but we giggled a lot when you asked if it was significant :)
"i know u can read this" — muu (Saor Gamers) for Watch Your Step
¬_¬
"I'm not even sure there are any blue arrows in this hall" — CSaur (Invaders) for Watch Your Step
"This 5 second cool down feels very limiting :P" — Steampunk_Sam (RGBeeGees) for Watch Your Step
"So much walking, damn you Ihunt team! DAAAAMN YOUUUUU!!!!" — Steampunk_Sam (RGBeeGees) for Watch Your Step
Let the hatred (and spam) flow through you!
"WE KNOW ITS BRAILLE WE CANT FEEL THE FUCKING LETTERS " — CodIsAFish (The A Team) for In the Land of the Blind, the One Handed Man is King
"IT SAYS GARBAGE, OK? IT MAKES NO SENSE. YOUR MORSE CODE IS BAD AND YOU SHOULD FEEL BAD!" — pingue (Step on snek) for The Perfect Desk Top Computer
Goood. Gooooooood! Let the hatred (and ultrasound) flow through you!
"No. No we can't . Get them you self " — theodonal (Yellows_r_us) for The Perfect Desk Top Computer
"THIS CANNOT CONTINUE" — Butcher (Fragsoc 1) for The Perfect Desk Top Computer
"More code are you fucking kidding me" — CodIsAFish (The A Team) for The Perfect Desk Top Computer
PERFORM OUR TASKS! DANCE FOR US!
Fragsoc, failing to find the start of the morse:
"Who's this he it speaks of?!" — Exilion (Fragsoc 1) for The Perfect Desk Top Computer
Yellows, unable to draw boxes:
"my box is 200 bloody px wide!" — chuff (Yellows_r_us) for The Perfect Desk Top Computer
"Take your 200x200 box and shove up your hard drive !!!! " — theodonal (Yellows_r_us) for The Perfect Desk Top Computer
Our reply: "Blow it out your zip drive!"
"titnerse" — pingue (Step on snek) for The Perfect Desk Top Computer
I don't know what that is but I wish I did.
"this radio sucks" — CSaur (Invaders) for The Numbers, Mason!
Yes, yes they did.
In addition, many people expressed their affection for Wizzo and his Mum:
"wizzo" — thedonut (Dulse Busters) for From the Shadows; "wizzosmum" — Manituo for Beyond the Veil; "wizzo" — Manituo for Beyond the Veil; "wizzosmum" — Al_Da_Best (Tigers) for A Garman; "wizzosmum" — Manituo for A Garman; "wizzoscum" — Manituo for A Garman; "wizzosmum?" — Al_Da_Best (Tigers) for Micro- and Macroscopics; "definitely wizzosmum" — Al_Da_Best (Tigers) for Through the Tubes; "wizzo" — Deathmendockx (RGBeeGees) for Watch Your Step; "wizzo" — fellastorm24 (Victorious Secret) for Watch Your Step; "wizzo" — CrouchingTiger (Invaders) for Watch Your Step
And their affection for us:
"penis" — Manituo for Beyond the Veil; "dickhead" — Manituo for Beyond the Veil; "cunt (says unspec)" — niax for Say What You See; "I'm so confused" — Taitenator (Crippling Depression) for Mixed Messages; "oh god screw you all" — Xik for Burn After Writing; "send nudes" — joejoe173 for A Pixelated but Impressively-Executed Texture; "fuck you" — joejoe173 for Through the Tubes; "cunts" — Rossiboi (The Mighty Mongoloids) for Through the Tubes; "dick" — fellastorm24 (Victorious Secret) for Through the Tubes; "dickfingers" — fellastorm24 (Victorious Secret) for Through the Tubes; "fuck this" — joejoe173 for Through the Tubes; "dick" — fellastorm24 (Victorious Secret) for The Trailblazer; "fucked" — iagowynne (Null reference pointer) for Rogueish; "send nudes" — beno754 (The Mighty Mongoloids) for Rogueish; "penis" — joejoe173 for Rogueish; "beth is a cunt" — joejoe173 for Rogueish; "fuck beth" — joejoe173 for Rogueish; "Cunt" — Lyxx (The Mighty Mongoloids) for Watch Your Step
Twelve people couldn't tell how many fingers I had and guessed "agliw" instead of "aglow" on The Calm Before The Storm. The Mighty Mongoloids were even sadder than Crippling Depression, saying "sadface" a total of 7 times. A lot of people thought we might have left our answers set to default: 10 guessed "password" to various puzzles. One person who shall remain anonymous got a bit confused with the letters in the Haptics puzzle
"kike" — [a terrible person] for In the Land of the Blind, the One Handed Man is King
I mean, I know we're horrible people but JESUS CHRIST!
And some people may have got a little bit more disheartened than we planned:
"i wish for the sweet release of death" — CodIsAFish (The A Team) for In the Land of the Blind, the One Handed Man is King
Though the most memorable guess has got to be the following, by ZeroG:
“Today, full of energy, Mario is still running, running Go save Princess Peach! Go! Today, full of energy, Mario runs Today, full of energy, jumping! Today, full of energy, searching for coins Today, keep going, Mario! Get a mushroom – it’s Super Mario! Get a flower – it’s Fire Mario! Goomba! Troopa! Buzzy Beetle! Beat them all! Mario is always full of energy and strong! [Spoken] The only one who can reverse the spell that has captured the Mushroom People is Princess Peach. But Princess Peach is hidden underground, in a far-off castle. Ah, the days of peace … if we could once more return to those days … to save Princess Peach and bring peace back to the Mushroom Kingdom, that is why Mario is on his journey today. Today, full of energy, Mario is still running, running Go and beat the Koopa tribe, go! Today, full of energy, Mario runs Today, full of energy, jumping! Today, full of energy, searching for coins Today, keep going, Mario! Get a star – become invincible! Quickly, go save Princess Peach! Lakitu! Blooper! Cheep Cheep! Beat them all! Mario is always full of energy and strong! Today, full of energy, Mario is still running, running He’s made it to the castle and gets fireworks! Lightly sidestepping the Hammer Bros. Show the last of your power, Mario! It’s been a long journey but it’s nearly at an end You’ve done it, you’ve done it! You’ve defeated Bowser! Princess Peach says, ‘Thank you’ Mario’s got a great big heart! Mario’s adventure is over for now, but Mario’s dream lives forever…”
Why the Witcher 3 is Good
3. May, 2016
What follows is not a review, not least because everyone who would care about reviews a year after the game's release has probably read them, and if not you may as well just go to [generic game magazine] and read theirs. Instead, I'm going to start from the point I arrived at while playing, which is that The Witcher 3 is probably the best single-player game I've ever played, and I therefore need to put that feeling into words and preserve them somewhere before I succumb to my own fanboyism and, I dunno, start bleaching and dyeing my hair Geralt-grey.
I should probably prefix this all with a caveat that I don't play that many story-driven games or RPGs, so it may be the case that a hundred other games did all or most of these things far better than TW3 does. (Feel free to make recommendations in that case). Still I don't think this is the case because so many big-name titles (ahem, Bethesda) fail on these counts, and because what I've heard from friends is so much more positive about this game than with others. And hey, it won Game of the Year for a reason.
Basically I want to examine all the reasons that made this game a success for me, why it left me feeling somehow adrift, a little... bereft after finishing it, having been drawn so wholly into the world the game constructs that I'd almost accepted it on some level as being real, as if its characters were important enough to feel real emotions for, as if, now I was wrenched back into reality, sitting on a sore bum in the same chair I'd been sitting for hours, something was now missing from my life.
It's the same feeling one can experience at the end of a fantastic novel or particularly moving film, and it only happens when whatever it was was so well made, so absorbing that it suppresses real life a little, takes over and sneaks in to your mind to the point where the realisation that it's now over is a genuine surprise, because how can something so real simply end?
Before I go further, a word on spoilers: I've tried to keep them to a minimum, but some inevitably remain. In particular, there is one pretty big point which is impossible not to spoil indirectly if one is to say anything about some parts of the game (this happened to me, as a matter of fact—if you've heard much about the game it may have happened to you too, without your knowledge). So, if you're going to play the game soon and want to keep your mind a perfect tabula rasa, it's probably best to stop reading; on the other hand if you're not going to play it at all, or not for a while, or if you don't mind a few indirect spoilers and allusions, read on. The more major spoilers I felt compelled to include are indicated; click to reveal them.
Bad aspects
First of all, I need to mention some things that weren't so good. This probably seems a bit weird considering my gushing opening paragraphs, but there's method in the madness. I'm going to say them first to get them out of the way, because I want to then be able to run away with an almost uninterrupted stream of disgustingly positive writing. I'm going to say them at all because they're pretty important: the game isn't perfect by any means, but almost all its imperfections lie in the gameplay. You'd think this would be the worst possible place for a game to have flaws, but the experience you have playing this game is rooted much more firmly in the story. In any case what you should take away is that these flaws do not detract from that experience.
The combat is very fun in Witcher: satisfying, fluid, skillful... but balanced it is not. If you start the game on a hard difficulty, you will be being shat on by every pack of level 1 wolves you come across. The first fight with a bunch of ghouls when you barely even know the controls is almost impossible; I think I had to try ten times to get through it. Even after managing a tough fight, you might come out with so little health and a marked lack of food or potions to restore it. You die. A lot. Discretion may be the better part of valour, but it's too extreme. Then, once you're level 20 or so, though, have levelled up your gear and, crucially, advanced and equipped some skills, you can more or less take on anything except the toughest bosses without breaking a sweat. Even enemies that out-level you significantly will fall because you'll have enough interruption, damage or defence to push through. This is a major problem.
On the other side of the coin, you sometimes come up against a critter who is just not susceptible to your particular build or strategy. Most bosses are immune to a lot of your "disruption" abilities—they won't stop attacking if you set them on fire or try to mind control them, for instance. If your strategy relies on something like this, you might find yourself for an unfairly tough battle.
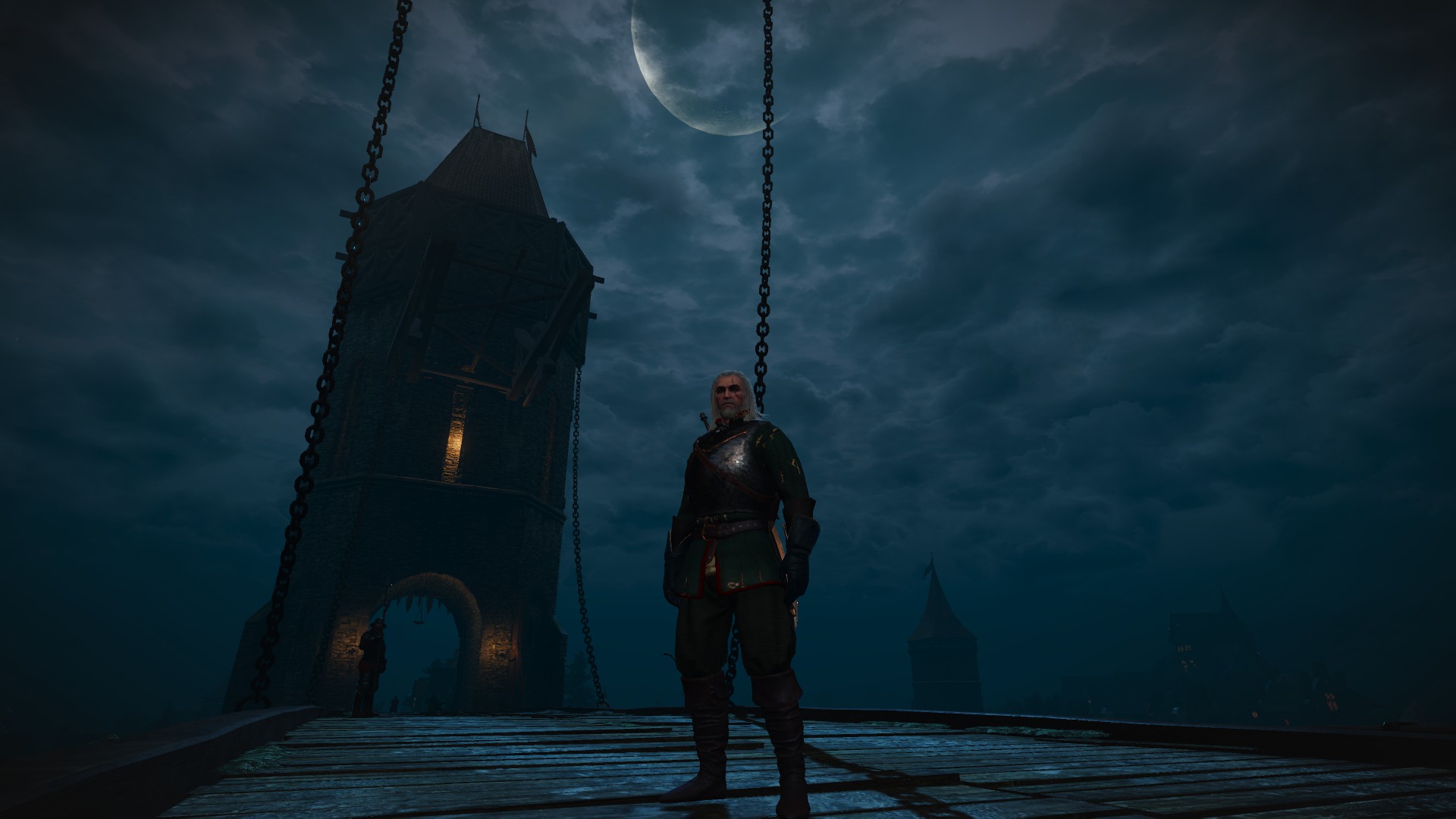
Your reward for these Herculean victories is invariably meagre: loot is lacklustre at best, and there's simply no reason for it to be. Most booty is just sold for cash since the best equipment is generally stuff you make yourself from special Witcher Diagrams, and everything else is basically just ingredients and minor buffs to your gear called runestones. You'll have enough of these to write a good-sized saga by the end of the game. This is exacerbated by the fact that potions and bombs never run out—you just meditate and, I presume, distill alcohol into sword-damage-juice in your urine, refilling your supply. This leaves the real scores as diagrams themselves, which enable you to brew more and better potions, and upgrade your swords and armour. But these are never dropped by enemies—they're found in specific locations and shops—so there's little reason to actually kill enemies, except for surrogate cash in the form of monster brains. Even your reward for completing contracts is a little measly (not enough to forge one of those shiny sets of armour, anyway) compared to selling your entire saddlebag full of rusty swords and ham sandwiches looted from the local bandits. Beside that, killing monsters gives you next to no experience points, so it doesn't level you up, either.
The whole levelling business is a bit weird, anyway. You gain most experience by doing quests—some questlines will advance you several levels on their own. But each quest has a recommended level, and doing it too late (more than 5 levels higher) will mean you earn about 2 XP from it. That's not an exaggeration: each objective completed might be worth just 1 XP. This isn't that big of a deal, and prevents you from overlevelling too much in a game where you end up the boss of everything anyway, but a traditional XP system where monsters and quests give you a fixed amount, but you need more and more to advance to the next level, would seem to soften the blow.
While doing these quests there is a bit of an over-reliance on the SpideyWitcher Senses doodad, too. This thing highlights all the interesting things nearby—not an inherently bad way of representing Geralt's supernatural senses, but probably fully 50% of objectives start with, "Use your Witcher Senses to..." and it gets a bit much. To be fair, it's very good that the game includes a style of objective other than, "kill this beastie," and "retrieve this trinket," or "talk to this dude," but nevertheless an alternative should have been found.
While using your super-smell, you can see what containers have stuff inside them, and where that cockatrice dropped his brain and other valuable body parts, which is definitely neat. However, trying to navigate to those delicious cockatrice craniums is sometimes frustrating, as Geralt always walks a little way in his current direction before turning to the direction commanded. If you're facing away from a monster drop, you can't pick it up, but if you try to turn towards it you'll likely end up facing a different kind of wrong direction, due to moving.
Other minor irritations are the various repeated elements: many NPCs share the same face, for instance—this is only so irritating because the faces are so detailed and varied that you can spot when it's been reused and covered up with a different moustache. At least each NPC with a cutscene could have been giving a unique face, though. Presumably this is a matter of the amount of work required to make models, textures and animations for every single face—their being so detailed must amplify that a lot. The conversations you overhear play every time you walk past an NPC—aka Nazeem Syndrome. You'll learn to hate that kid who sings "Emperor Emhyr is snoring" before you leave White Orchard. Finally, while the animations are all impeccably done, some of the conversational animations, particularly hand gestures, are just played way too often. You get to recognise that weird "I'm explaining something big" gesture coming from a mile off.
The Rest
Story-telling
The most important strength of TW3 is its story-telling. You rarely get a story so engaging and complex, never mind in a video game, but in most films. In a way, this is unsurprising: a two-to-three-hour film cannot hope to convey such a complex story as can be told on your journey through a hundred hours of gameplay, even with a lion's share of that play taken up drinking up the gorgeous landscape you must ride across, and slaying the monsters you're paid to deal with.
But while time is a key advantage that a game has when telling a story, any gamer knows that most games have simple stories that are enough to hang the mechanics on, but in the end is just like so much washing line.
One of the first aspects of TW3's strength you encounter is the structure of its main quest. You are searching for your adoptive daughter, Ciri, who also happens to be sought by a whole bunch of other people (she's very special due to her birth and blood, you see). There's nothing special here, but as the story advances, you are given the information you ask for by the people you meet, and each time another piece of the history of her journey through the land is revealed, you get to experience it first-hand, playing as Ciri.
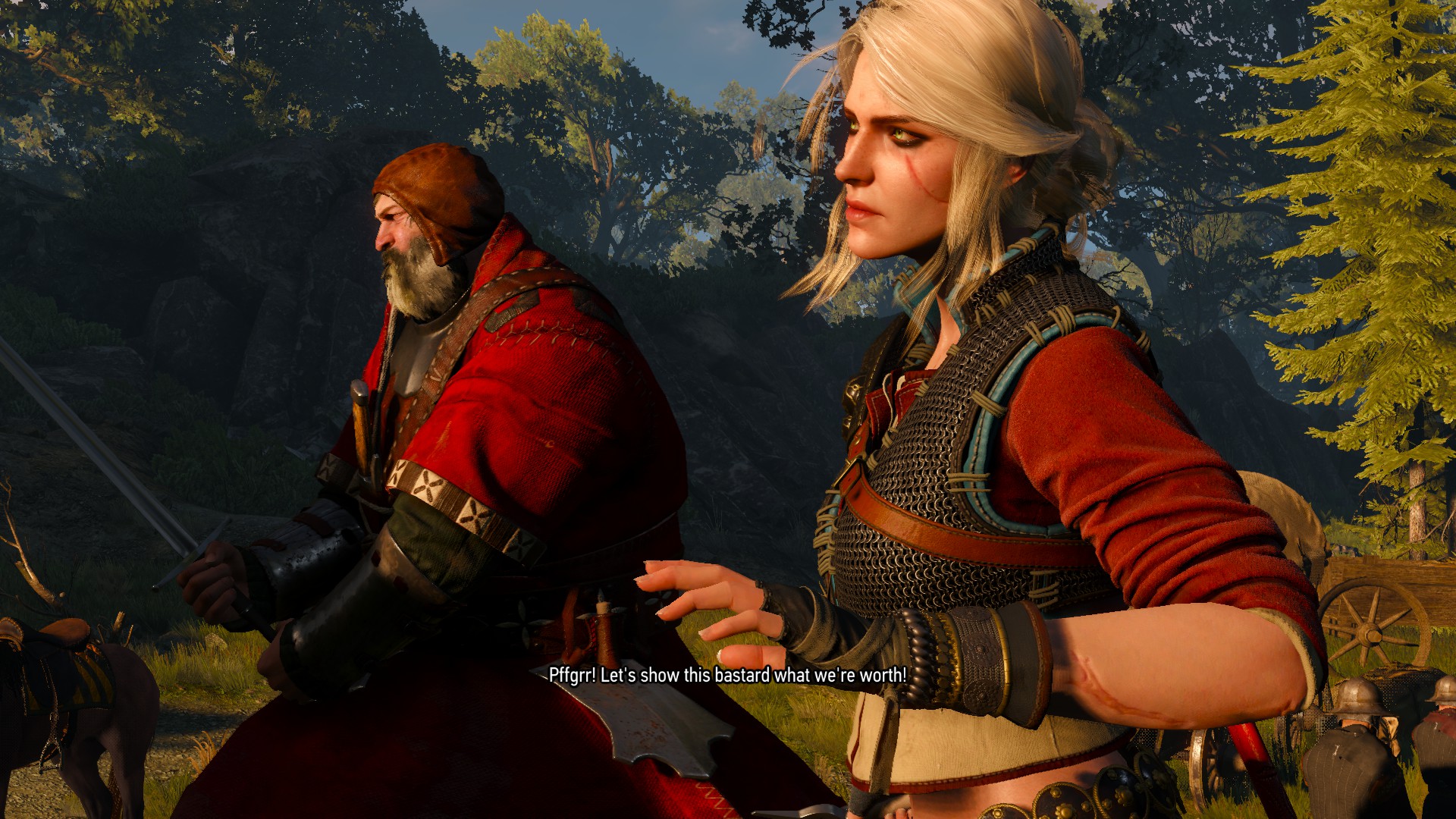
This provides some exciting gameplay variation because Ciri's abilities are very different from Geralt's, but for the story, this attunes you to Geralt's mentality: it is clear he cares very deeply about his ward, and so for him, when someone is telling him of the dangers she faced, it's as if he is reliving them himself.
This is crucial because the success of the story depends on you caring about its characters in a way I rarely do in any work of fiction. Interactivity is key, here: you play to be Geralt, making his decisions, choosing his words and actions. This moves you to think more like him, to care about the things he cares about. Time is important again: it's difficult to grow to care about a fictional character, but it's harder still if you only have a couple of hours to get to know them. But then, with characters like this game has, maybe this is less of an issue. Ciri's mixture of strength together with her relative lack of experience makes for a delicate balance: you want to help her as a daughter in need, but not to be over-protective of someone who knows she is powerful, who needs to be allowed to become fully independent.
This comes to a head when you finally find Ciri. And she's dead. Or rather, she appears to be—she is revived by a fairy light thing, but not before Geralt has collapsed, head in hands, and is crying into her lifeless shoulder. The whole sequence is beautiful, painfully beautiful. The only issue here is that this is the point which is so easy to indirectly spoil—any mention of Geralt doing anything with Ciri implies that she must live beyond the point at which you find her.
The game abounds, though, with people to care about. The story of the Bloody Baron is a tragic and complex such person: a deeply flawed man whose friendliness can't hide his terrible faults, yet whose situation begs for sympathy. Your various love-interests all have their own strengths and resolve, yet are vulnerable in their desire for intimacy and human contact; the interaction of their own agendas with Geralt's desire to get laid makes for a humorous ballet, that always ends up feeling somehow comfortable, which is an aspect of real relationships often missing from fictional representations. Triss, an old flame (literally—her preferred offensive spell is the classic fireball) but since apparently spurned by Geralt, clearly misses him. How you treat her affects several other interactions down the line, but what's clearer is your desire as a player to treat her kindly and not cause more suffering. How you do this (or if you don't, and rub it all in her face) is up to you.
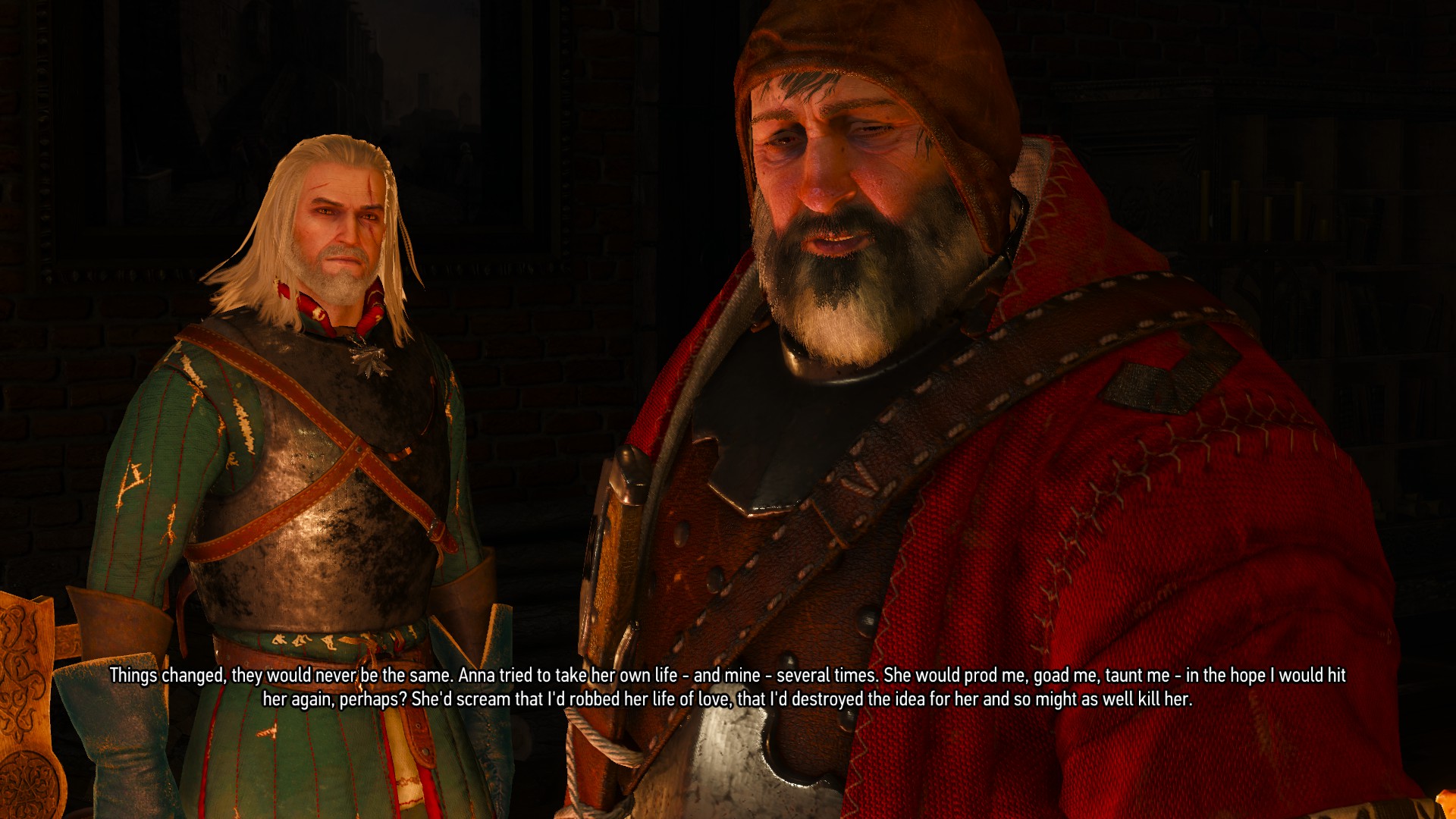
In all of these developing stories and relationships, the writing is impeccable. In contrast to some of the overheard NPC-conversations, the dialogue never sounds off or unrealistic, and you never pick a dialogue option only to realise what you actually say was totally ridiculous. In fact, the opposite is true: the options are designed to make it clear what choice you're making or expressing, but what you actually say often bears no resemblance to the option (to the extent that sometimes you say nothing) yet this not once goes wrong. You can trust Geralt to say something good, evenespecially if you always choose the most sarcastic option. (Sarcasm is frequently an option: you should definitely always pick it; it will definitely 100% never go wrong. Well, it will never go boring.)
Layered on top of this is absolutely fantastic voice acting. The main characters aren't even the stars, here, though I was pleasantly surprised that Geralt avoids the Surly American Hero stereotype to a degree—the sensitivity in his voice when talking to children or scared creatures prevents this. But there's the aforementioned baron, whose gruff Liverpudlian baritone perfectly matches his outward appearance as a large, tough leader who loves a drink and a fist-fight. We are therefore all the more off-guard when his sins and melancholy are revealed. Keira Metz is an old sorceress friend of Geralt's, whose voice carries a fantastic tinge of haughty command alongside a mischievous humour and affection. Then there's Avallac'h, a character you don't meet until much later, whose motives are unclear but whom you nevertheless need to trust. His voice is the essence of calm—assured in himself and his task. I don't really have anything to say character-wise, but his voice is so rich, I could listen to it all day even if he were reading party-political broadcasts for the BNP. The only niggle with the voice acting is a perennial one: when a character cuts another off there is always a small but noticeable pause where one sound stops playing and the other begins. Obviously voice actors are recorded in isolation but you'd think games would be able to fix this by now!
All these voice actors are chosen with a variety of accents that warms the cockles of my soured, English heart. It wasn't until I started watching Game of Thrones that I realised I missed hearing British accents in my blockbuster TV shows, and with TW3 the same feeling of slightly-embarrassed (because really I should be able to enjoy a film, show or game just as much regardless of where the voices come from, right?) glee to realise that they not only have English accents, not only Received Pronunciation, no! They also have voice actors (or accents) from Liverpool, Yorkshire, Wales, Scotland, Ireland (I think both Republic and Northern), the West Country, the South-East and probably other places besides. While this did lead to one rather sad interaction with an art collector in an auction house—the speed with which he switched from West Country to Yorkshire potentially having ramifications for British cross-country rail operators—pretty much everyone else is flawless.
But that is a rather personal and secondary consideration. Returning to more sensible things, the acting in general represents a major milestone in gaming. The character animation and directing is (with exception of some of those weird hand gestures) incredibly well done and, above all, subtle. This is the first time I noticed characters in a game actually exchange glances, for example—wordless, meaningful looks. They might smirk, or roll their eyes, or grimace, or convey any emotion. Pay attention, Bethesda: it's no longer enough for characters to stare at you, gormlessly relating the awful loss of her family with an expression you might have if you zoned out while watching a particularly unexciting episode of Countdown. In Witcher it's there and it makes a massive difference in the amount of emotion you feel from a scene. Correspondingly it allows you to engage with those characters on a much deeper level, just as you can when watching a well-acted film, but with all the advantages the game and its format bring. Of course, it's not on the level of real actors just yet, but games are getting there, and game developers are very definitely aware.
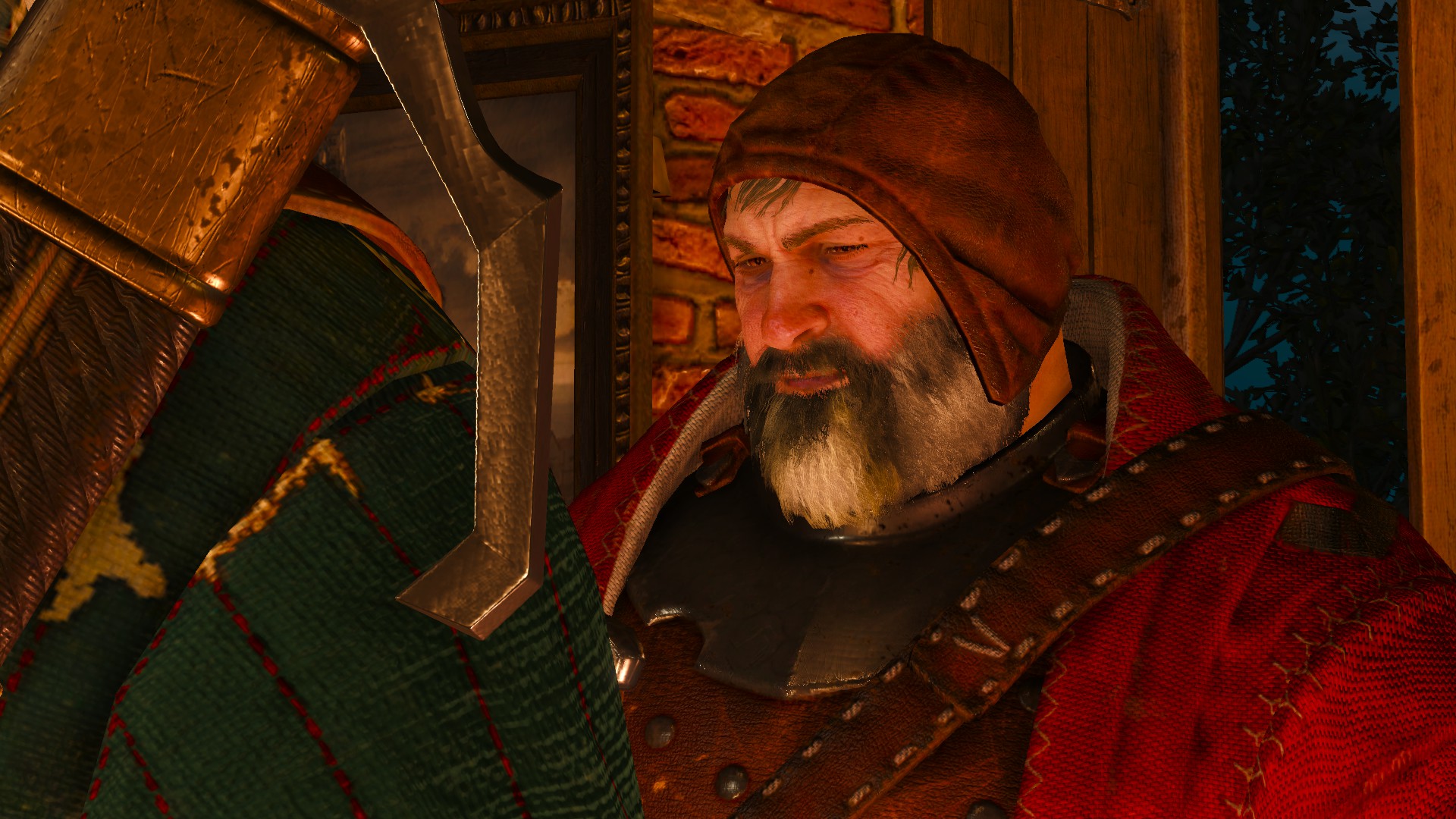
One example of fantastic acting occurs when you can choose to let Ciri vent her anger—in fact one of the cases where a dialogue option leads to you saying nothing. If you take this option, Geralt pretends to read some bits of paper, while deliberately knocking a bottle off the desk, smashing it. He pretends to have done it by accident, but he and Ciri share a look and she starts to smile—then mimics his "accidental" violence, and chaos ensues, all while Yennefer observes, trying to look disapproving.
Engagement
The success of the story-telling leans in no small part on your engagement to the story by giving you interesting things to do and see through quests. This is something that the Witcher series is famed for, and it does not disappoint: on multiple occasions, I just gaped at the screen at what had unfolded, or grimaced as I had to make some awful choice.
An over-arching theme of the game is choosing the lesser of two evils. In these quests, there is often no right answer: you can choose to assassinate a king or not—which is right? Which will cause less suffering? And later on can choose whether to stay true to an idealist ally, or side with someone who sold out your cause for a negotiated peace—the same questions are just as hard to answer. Games have been able to let you choose between being a White Knight and an Evil Bastard for a long time, but it's much rarer that you have a decision which you can't just make based on your D&D character alignment. This is crucial, because it forces you to think about your choice then encourages you to live with it. You could just reload if something unexpected happens, but you're discouraged from doing so: after all, you made the choice for a reason, so it would be going back on your own principles which you used to reach your decision.
As the game progresses, you realise that the philosophy of the game is choices with consequences, too. In Tower Full of Mice what you do could result in an awful fate for one or more people, and it's not obvious what to do. But you know the rules of the game by this point, and so it seems fair, whatever outcome you get. The consequences you anticipate force you to consider your options carefully, to try to pick carefully—the game forces you to engage with it, by making you suffer a litany of death if you breeze along.
Many quests suffer from being part of a classic RPG-train of quid pro quo—you need to find Ciri, but the Baron won't tell you where she is unless you do something for him, but you can't do that without the help of Zoblog the Great, who wants you to fetch a MacGuffin, which... you get the picture. However, while this does sometimes get a bit tedious, it's averted to a large degree by involving tasks other than simply "fetch this bauble" or "kill ten rats skeevers ghouls." Often your task is to tell someone something, or ask them about something, or look for someone—tasks which seem a little less shoehorned into the framework of the game than your classic Skyrim, "if you want me to do this for you, please help me by going into this dungeon and killing some draugr."
To a large extent, the fact that you care about the characters means you care about the outcomes of the quests, too—so you want to help Triss rescue her mage friends, and you're equally motivated to work out with Yen whether your mutual attraction is natural or the product of magical interference. It matters little if you're being sent from pillar to post (from Pellar to... Goat?) if you actually care about the consequences of going to those particular architectural features. These two quests are not in the main questline and are entirely optional—but they cry for your attention nonetheless. They have big consequences for the characters involved, and increase your understanding of and attachment to them.
Many quests in TW3 require no combat and even less interaction: they're basically cutscenes with intermissions to keep you awake. But this is completely unnecessary, because you're generally glued to the screen watching events unfold in rapt attention. Any dribbling on your keyboard is because you're gaping at what happened, not because you've dozed off. A notable example is Va Fail Elaine, where an event that's been building for ages finally pays off and you get some concrete progress. All you have to do is watch and listen (to a panicked maybe-human creature essentially being tortured) and administer three potions.
Art
Some people like to debate whether video games are art (or, perhaps, "Art.") I find this a weird debate because it seems so obvious to me that they are. Nevertheless, many games are not very good art.
TW3 is very good art. As a work of fiction it is complex, nuanced, and draws you in more effectively than any film or book because of the nature of the medium, well-executed. As a piece of visual art, it is impeccable: for a while, now, computer games have been capable of producing such beautiful vistas as to rival any real landscape. When the sun is setting in White Orchard, or rising through early-morning rain on Ard Skellig, one is moved to pause from the tawdry business of hunting monsters, from the humdrum of rescuing your lost and only daughter to take in the beauty and atmosphere.
Remaining visual, this is the first game where I've actually noticed the camera in a positive way. Most of the time in a game when you notice the camera, it's because it got stuck in a wall, or is helpfully staring up your arse instead of giving you a view of whatever flying beastie is trying to relieve you of your limbs. Not here: every significant piece of dialogue has the camera move around as if directed by a proper cinematographer. Probably it was. The angles, cuts and pans all contribute to the telling of the story just as they do in a quality film production.
The animations don't just add to the acting and realism of the characters, but to the impact of the game visually: significant boss-battles end a bit before you actually deplete their vitality; the last few strikes are out of your control but brutally and beautifully choreographed. Your rage at defeating someone who would harm Ciri is palpable and gruesomely rendered, your desperation in dispatching a closely-matched foe is stark. The gameplay animations are themselves excellently done: Geralt is nimble on his feet, dodging deftly, at your command, to the side of blows, leaving him in position for an attack at an opponent's undefended back or side—fluidly transitioning from defence to offence. This lends combat itself its own flowing beauty (in spite of problems mentioned above). Nevertheless, no in-game animation is as perfect or detailed as those custom-made for the cutscene at the end of the battle, and these are a welcome addition.
The music must be mentioned at this point. Game music is something that has been incredibly good for a while, TW3 being no exception. It's very gratifying that this aspect of games has reached this level, where it not only enhances the atmosphere while playing, but is well worth listening to on its own. Somehow I feel like game soundtracks are easily dismissible, since you rarely pay much attention while playing in most games, but this is frequently no longer the case.
One thing the soundtrack does suffer from is repetitivity: because each piece is tied to a particular location, you are very apt to hear the same tune repeated over and over, and no matter how beautiful a piece is at first, it begins to lose its charm when you've been wandering the Fields of Ard Skellig for a couple of hours hunting down harpies. This strong tie between situation and track is not without its benefits, though. Like all major locations, the village of White Orchard where you start the game has its particular music, but due to its nature as a bit of a training zone, you can easily not return after you leave. At one point I returned there, hunting for alchemical ingredients (Arenaria grows there in abundance, and you need a lot of it for advanced potions) and the music immediately recalled those first hours of gameplay, first forays into the world, first battles and victories.
Likewise, the driving, whirling motif of the Ladies of the Woods instantly recalls their twisted likenesses and disturbing movements; the gorgeous, soaring notes heard on Skellige call on memories of wide fields and plunging cliffs; while the thumping, forceful rhythm accompanying the eponymous Wild Hunt and its generals brings memories of desperation and the rage of battling hated opponents. Meanwhile, the music that plays in the villages and countryside of Velen—most of the map outside Skellige—evokes in itself a simple peasant life, interrupted and cut through by the bitterness of war.
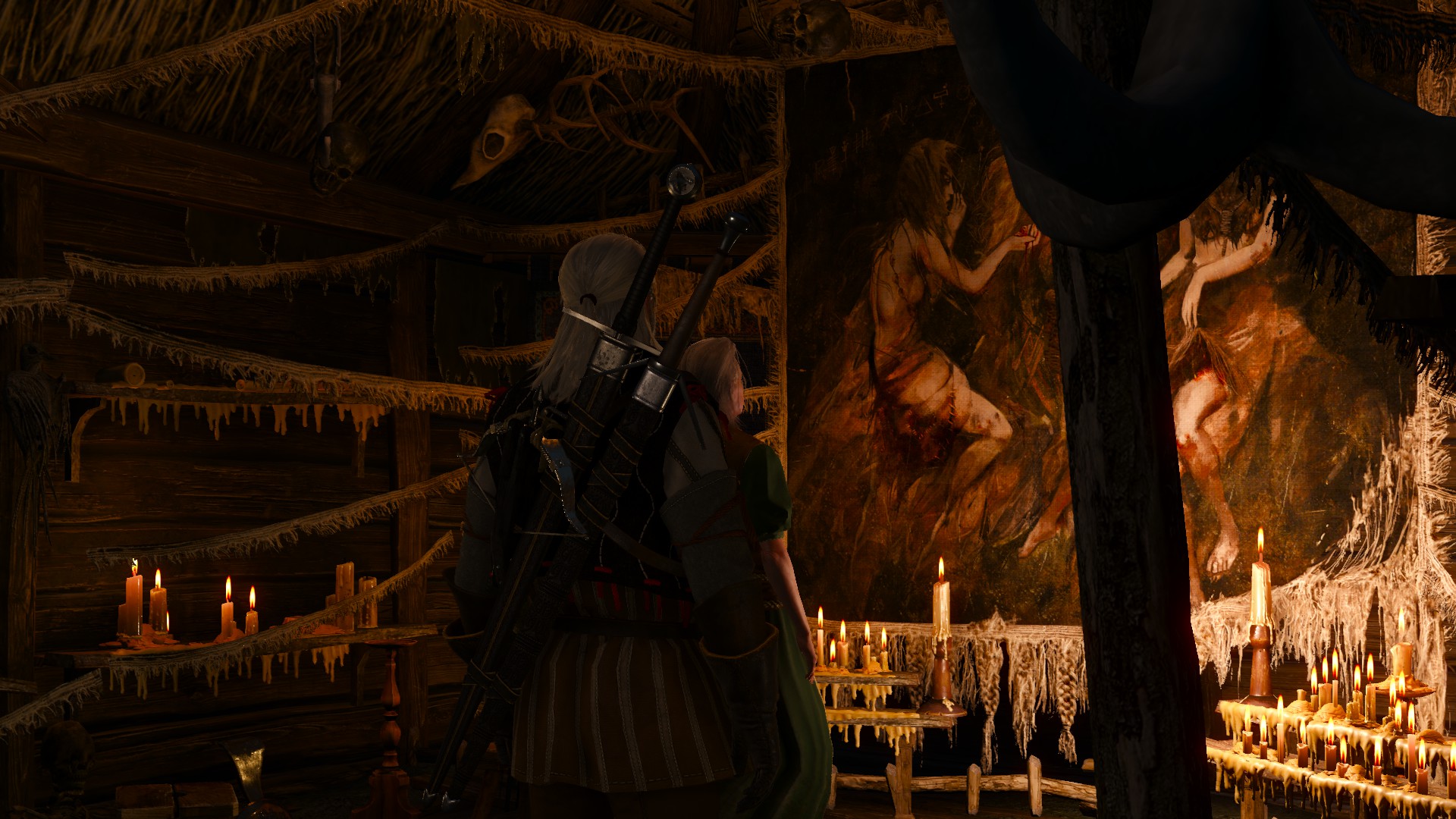
But this is only one layer of the artistry of the game. It's easy to point to technical features and concrete bits of media that are artistic, but the game's true success goes beyond that:
Emotion
Some people[who?]—shut up, wikipedia! I guess would say something is only art if it gets you to feel emotion. Whether or not that's true, perhaps it's easier to agree that playing on one's emotions is the sign of good art. And the breadth of emotion felt in TW3 certainly elevates it to that level. This would be impossible were it not for your engagement with the story and its characters, because you have to believe in those things, become invested in them, feel like they matter, if you are to feel anything about what happens to them.
Now, games are pretty good at getting you to feel some kinds of emotions: happiness and satisfaction, for example, at overcoming obstacles—or frustration at the opposite—are common and mundane. Laughter is fairly easy to produce through humour (which doesn't hinge on character attachment)—something which abounds in TW3. Let me digress in that direction a little:
Comedy is around every corner in this game. Geralt himself possesses a certain kind of dry wit, often arrogant, invariably sarcastic, that produces many a cackle. One quest sees you trying to lead a goat back to its owner by ringing a delightful little bell at it, whilst calling its name: "Princess." The whole scenario is ridiculous, as our hunk-of-mutated-man-meat protagonist walks through the forest tinkling this bell and crooning "Priinceeess!" But best of all is that Geralt knows it, and detests every minute. Until the end, when he seems to be forming a beautiful sort of bond with the animal.
Before this there is an encounter with an exquisitely proper chamberlain, who only addresses Geralt as, "the Gentleman" yet who seems to wield his politeness as a weapon to express his disdain. The feeling, of course, is mutual, expressed in turn by dripping sarcasm. Every troll expresses hilarious insights like, "Bart no minute got. Bart rocks got." Dijkstra's verbal swordplay with Geralt cuts just as well as the latter's blades, sarcasm that leaves me in stitches every time. (Dijkstra, incidentally, is another character voiced by an absolutely impeccable actor)
Later, you have the opportunity to get absolutely trolleyed with your fellow witcher buddies, and what follows is purest comedy gold, culminating (potentially) with one of your chums suggesting to call in some sorceress friends so they have some female company or, as he puts it, to "SUMMON THE BITCHES!" Funny enough, perhaps, for a tough, experienced monster-hunter to slur out words more suited to a freshers' party, but much more so since if any of the "bitches" in question heard him, he'd probably be spending the rest of his days with his body a little more dispersed and a little more crispy than is strictly ideal for remaining alive. All this jocularity manages to come with a keen human quality that sets aside from simple comedy: it's the realistic humour that happens between people who know each other well, care for each other, and are comfortable with one another.
This is what sets the humour of TW3 apart from mere jokes and funny lines: it's the kind of humour that I love Firefly for capturing so well—realistic and unforced, completely at home in spite of (or perhaps because of) the uncompromisingly cruel world. It doesn't end there, though. Because you feel such care for the other people in this story, you empathise with them, share their sorrows—such as in the case of the Baron. You feel anger at the Wild Hunt for trying to take Ciri, and occasionally you feel happiness, as when a fellow witcher can find a girlfriend and move away with her.
Revulsion is easy to evoke in a game, especially with modern graphics, but the developers seem to have a certain knack for the vile and disturbing. The botchling (in other words, just a casual demonic miscarried foetus), the Ladies of the Woods, the Pesta (and, potentially, her blood-curdling, repulsive kiss... eugh) are all awful, sickening, deliciously horrid.
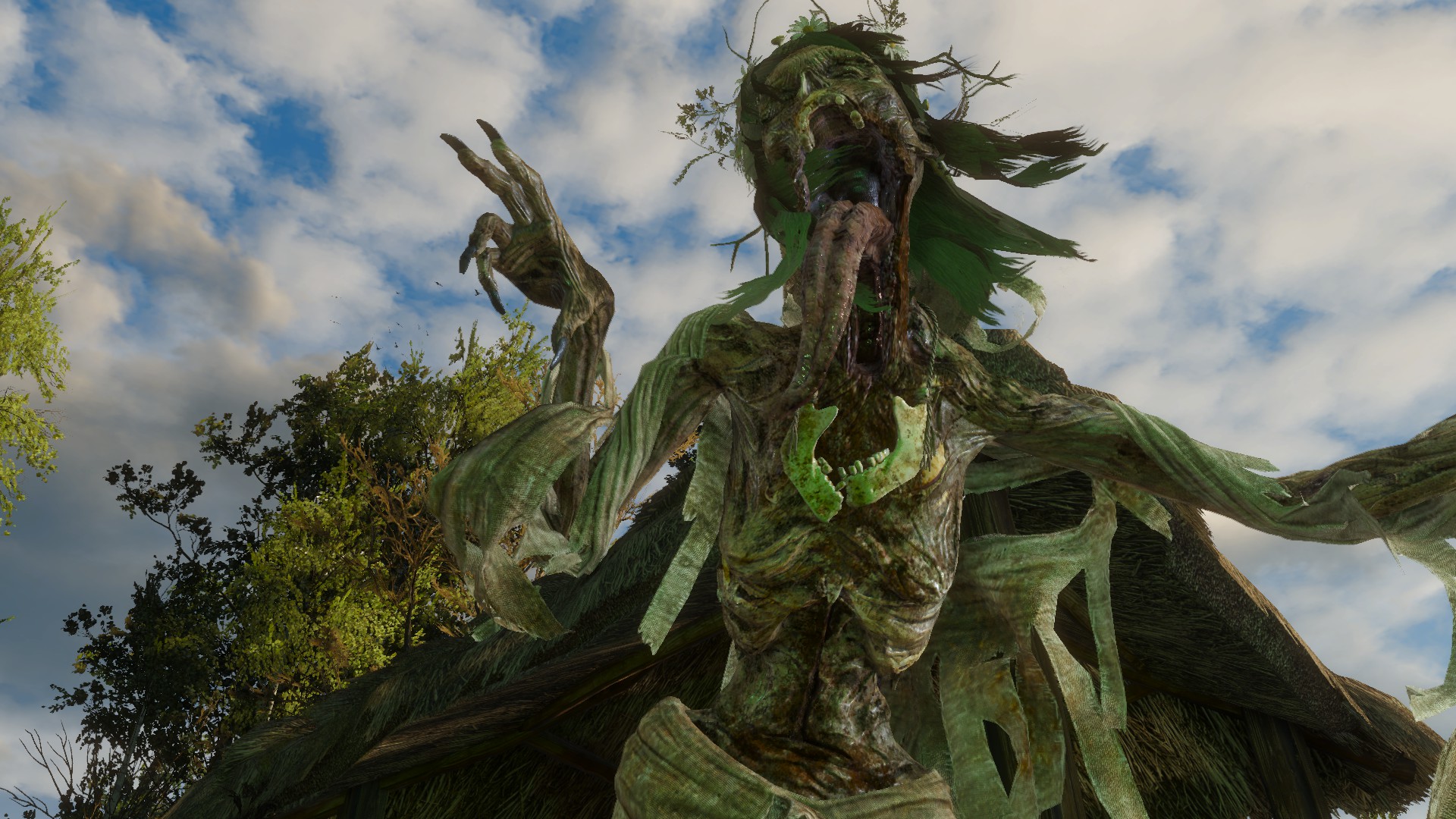
Perhaps one of the most forceful emotions is sadness. I love a good tragedy, and so much of the world of The Witcher is tragic. In a game where so many choices have no right option, you are faced with it frequently. Throughout the main quest we find sorrows great and small—the Baron's story has already been mentioned; turning down potential lovers results in heartfelt reactions to your rejection; Tomira's simple story of her fall-out with the Temple—all are poignantly rendered and acted.
Nowhere is this more evident than the ending. There is more than one ending, but all of them have some sadness to them; all brought tears to my eyes. One is perfectly bittersweet—a victory, yes, but also a loss of another kind. Another is almost harrowing, and ends with Geralt apparently waiting to die. After completing the game I watched all the endings on youtube. Somehow it seemed like they all happened, because they all could almost have followed on from my game. The sorrow of what might have happened is felt as keenly as the experience of what did happen.
Specifically, there are three endings, depending on how you treat Ciri in the latter part of the game. If you treat her poorly: being over-protective, not cheering her up, for example, then she will just die. The epilogue is Geralt returning to the remaining, living Crone, who stole Ciri's medallion. Upon retrieving this last memento of his adoptive daughter, he retreats to a cabin in Crookback bog, as monsters close in from all sides. It's horrifying! You spent over half the game looking for her, found her, managed to help her save the world and now... all you have left is this necklace. Geralt wants to die, and so do you.
Alternatively, if you treat her well, return with her to the Emperor, her real father, who then survives the war and political machinations, the epilogue consists of you two spending some beautiful father-daughter time together. Yet something is clearly getting at Ciri, and it's not long before you find out: she's been groomed to be the Empress, and your time together is cut short by a retinue come to ride with her back to her father. You may well never see each other again. If anything, the loss is even greater than if she'd died: it's absolutely, crushingly soul-wrenching.
Even the "good" ending jerks some tears, because the first scene after saving the world, you return to the Emperor's palace and tell him that Ciri is dead. There's no hint that you're actually lying until a few minutes later. Here was my reaction on IRC:
[02:21:10] <Fish-Face> wha [02:21:15] <Fish-Face> I got the sad ending DDDDDD: [02:22:18] <Fish-Face> I am pretty gutted [02:26:44] <Fish-Face> AHHHHHHHHHHHHHHH [02:26:47] <Fish-Face> FUCK YOU GAME [02:26:50] <Fish-Face> OH MY FUCKING GOD [02:26:53] <Fish-Face> DON'T DO THAT TO ME [02:27:06] <Fish-Face> LIUHAGEILUHWRGLHE:OEWIJGHLIWEHG:OFIJEQLHBGQE J:OIFELKGLHRW
I stand by that.
And then, with a deep intake of breath, I'm back in the real world. I've been sitting in the same position for too long, and it's far too late. Yet I can't sleep now; the events of the past few hours are still spinning in my mind—the victory and loss, joy and sorrow are still mingling and whirling there, along with all the other events and emotions from the play of the past few months. So I lie in bed and think about what an incredible experience it was and mourning—not so much for those fictional people whom I saw die, but for the end of the adventure: the journey which absorbed me for so many hours, drew me in, flung me round and spat me out again. This is its own bittersweet feeling: the story is over, but I wouldn't be wishing it weren't if it hadn't been so good.
Talk notes: ULTIMATE POWER
17. January, 2014
Here are the (slightly expanded) notes from my talk about Ultrapowers.
16 roughly distinguishable colours
7. January, 2014
If you're colourblind like me, distinguishing colours is pretty tricky, especially if they all have to be dark and not look totally horrible. This comes up if you use colour to help tell apart nicknames on IRC at a glance, where the more colours you have at your disposal, the better.
After a few hours of fiddling, using python to generate the colours and test output, I came up with the following colours:
#892929 #c36d3a #897129 #9da030 #598929 #37ac33 #227236 #2c956e #257e7e #3787b7 #304ca0 #503dce #52257e #bc3dce #a03084 #b73767
Here's a graph of them:
Angle (0-360°) represents hue and distance from the origin represents value in HSV colour space; all points have saturation 70%.
I started with 16 points distributed with 4 in an inner circle (V=0.5), 4 in an outer circle (V=0.8) and 8 in a middle circle (V=0.65), then played with the precise hues and values. I ended up darkening the greenish colours since they appeared too light otherwise. I also had to manually push around certain similar-looking colours.
Feel free to try them in your IRC client or for some other purpose.
Search Quassel Logs
6. January, 2014
I use quassel as my IRC client. Before that, I used irssi , and before that I used XChat and a bouncer, all in the name of not missing anything that happens on IRC. Arguably this is all a bit silly, since not that much actually important happens on IRC, and in any case if you miss it, you can just ask someone else to fill you in. But nevertheless, I find staying on all the time worth the hassle of finding the right client and currently I find quassel to be the best solution — it has a proper UI unlike irssi, so it's easy on the eye and has keyboard shortcuts, etc; it doesn't require a nasty bouncer with all the ugly scrollback that entails; it's actively maintained; and it has a decent Android client.
But the disadvantage is that, after accumulating all of those lovely logs, it's really hard to search through them. Traditional clients save their logs to text files on your hard drive and you can just search them with any text editor or more usually, grep
. But quassel's logs are stored in a database and crafting the correct SQL query by hand every time is a pain, and crucially quassel currently has no means of searching logs that aren't loaded from within the client. You can use the PHP-based quasselsuche but that requires installation and going through webpages and such, and I wanted a lighter solution. In-client log searching is a long-term feature request for quassel that's sure to be implemented at some point, but until then, enter quasselgrep!
quasselgrep
is a command-line tool in the spirit of grep
which allows you to easily search quassel's logs. Documentation is available on the github page/in the README and should be fairly easy to follow, but in brief: You run quasselgrep
on the server where quasselcore is running (or through an SSH tunnel), either running queries directly, or in server mode so quasselgrep
clients can connect to it — useful if you're hosting a multi-user quasselcore.
An example query would be:
$ quasselgrep -b #quassel -n Fish-Face -t yesterday quassel
Which searches the channel #quassel for lines by me, sent yesterday, where I mentioned quassel.
quasselgrep
is still under development and needs bugs finding and features adding, but should be quite serviceable. Please report/comment on the github page!
NetHack statistics
27. October, 2013
I've recently been playing NetHack on the nethack.alt.org server and was wondering how likely you are to ascend depending on how long you survive. If you don't know, NetHack is a notoriously difficult game, and few people ever beat it (or "ascend" as it's known.) However, the greatest difficulties occur in the early game, when not just mistakes will get you killed, but also the foibles of the Random Number Generator (or the Random Number God as s/he is sometimes called.) So with the help of nethack.alt.org's extended log, documenting over 2.3 million plays of the game, ipython and matplotlib, I cooked up the following graph:
This graph shows your chance of ascending based on how long you survive in the dungeon, clearly demonstrating that the longer you survive, the better your chances. Notice though, that it never gets that certain: no matter how long you survive, it's possible to fall from glory (or, "Splat".) As the turns go on, fewer and fewer people have survived without already ascending, and the graph gets jagged and unreliable.
But what exactly is happening to our 2.3 million adventurers over the 100,000 turns graphed here? Time for more graphs:
On the left, we see the number of players dying, quitting or ascending on each turn, on two different scales: Firstly at a large scale (but still not nearly including the vast numbers of people who quit or die immediately!) for the first 5,000 turns and then zoomed in up to 50,000 turns. Both graphs have been smoothed. Note that of the 920,000 "deaths" on the first turn, almost all are due to escaping the dungeon.
On the right, we look at how many players there are of each status (alive, ascended, dead, quit) after a number of turns. On the first graph you can see that the tiny quantity of ascensions barely even features, while in the zoomed-in graph you can see how the ascensions begin to accumulate. Since many many people quit on the first turn (either by #quitting or escaping) we have excluded them from these graphs.
Here's the code I used to gather statistics on the xlogfile:
import re from numpy import * def movingaverage(interval, window_size): window = ones(int(window_size))/float(window_size) return convolve(interval, window, 'same') l = 100000 r = re.compile(':death=(?P<death>[^:]+).*:turns=(?P<turns>[0-9]+)') total = 2361141 #Total number of records with extended data available def parse(): global dies, quits, asc, alive, alive_asc, alive_asc_quit dies = [0] * l quits = [0] * l asc = [0] * l for line in open('xlogfile.full.txt', 'r'): m = r.search(line) if m: turns = int(m.group('turns')) if turns >= l: continue if m.group('death') == 'quit': quits[turns] += 1 elif m.group('death') != 'ascended': dies[turns] += 1 else: asc[turns] += 1 cumtotal = total - dies[1] - quits[1] alive_asc_quit = cumtotal - cumsum(dies[2:]) alive_asc = alive_asc_quit - cumsum(quits[2:]) alive = alive_asc - cumsum(asc[2:])
Now back to 'hacking!
Mingle 2013
25. September, 2013
Here are the slides for my talk at Mingle 2013, 26th of September at @Bristol.
Cardinality
19. May, 2013
It's time to make a post about MATHS.
Most people who know some mathematics know that there are infinitely many natural (whole) numbers. It's easy to see — you can always add one to a number and you get a brand new one. Clearly this also goes for many other sets: there are infinitely many even numbers, odd numbers, rational numbers (fractions) and real numbers (decimals). But one of these sets is not like the others — it is bigger than the rest!
Most people (unless they have inside knowledge) will think this is either obviously true or obviously false, and they will both be wrong! The first person will think that there are obviously more natural numbers than evens, more rational numbers than natural numbers, and more real numbers than rational numbers (since every fraction can be written as a possibly repeating decimal, but there are irrational numbers, like .) The second will think that they are all just infinite, and therefore the same size — if you add something to infinity, you still have infinity.
The first person is right, to a point. However in mathematics once you get to dealing with infinite sets we need to pin down what we mean by "size", and the concept we landed on is "cardinality." The upshot is just that two sets are the same size if the elements of each set can be paired off with each other, so that all elements of set A have one and only one corresponding element in set B, and vice versa.
Thus there are as many natural numbers as even numbers, because we can pair each number with the even number
. It's easy to see that there is one, and only one,
for each
, and one, and only one
for every even number — since an even number is a number in the form
:
What we've done is exhibited a bijection between the set of natural numbers, , and the set of evens,
. A set that is in bijection with the natural numbers is called countable. The link shows how it is possible to prove that the set of rational numbers is countable.
So, up until now the second person was correct, but no longer. Cantor proved in 1891 that there are more reals than there are naturals — that the set of reals is uncountable.
The proof is quite simple. Suppose is countable, and we will find a contradiction, so what we supposed is impossible, and
must be of a different cardinality than
. (And, since
, it is clearly bigger!)
To be countable, there must be a bijection — a function from
to
pairing off the elements with each other. We can illustrate such a function as follows:
Now we have to imagine that this table goes on forever down and right, since there are infinitely many s, and each
can be infinitely long if it is irrational, but that's OK. This table just illustrates what part of one possible potential bijection would look like, and we are about to argue about any candidate — it's important to remember that whatever
we try, this argument will still apply, whether we try to modify it slightly, or pick a completely new one. It also doesn't matter that we only showed numbers between zero and ten.
Suppose we had indeed paired off everything, so that every real had its , then I will show how to find a new real number,
. The idea is that we will show that
does not appear anywhere in the table, and hence
will not be a bijection (if it were, then every possible real, including ones we cook up in fanciful ways, must be listed.) Then, we will have a contradiction, meaning that
is not countable.
The contradiction follows from looking at the diagonal of the s. So, look at the first decimal digit of
— above it is 0. If it's zero, then the first digit of
will be one, and otherwise it will be zero. So for the above table,
starts
. Then, look at the second digit of
. Do the same, so
now starts
. Continuing in this way, we get
, and the
th digit of
is
if the
th digit of
is
, otherwise it is
.
Now, if were indeed a bijection, as we want it to be, this
must be listed on the table somewhere — every real number is. But it is quite obvious that
does not appear, because it is different from
at position 1,
at position 2, and so on — it is different from
at position
!
And that is the contradiction. Any possible bijection — one-to-one pairing — of the natural numbers with the reals has this problem, so no such pairing can exist. Hence there really are more real numbers than there are natural numbers.
Now, some people will be entirely convinced by this while others might have objections. The first common objection says, "why couldn't we just add this new number to the list?" But remember, the argument above works with any possible listing, so just adding a number (or infinitely many!) to the list won't help; we can run the same procedure and find a missing number still.
The second common objection is that the notion of cardinality must somehow not be fit for purpose. After all, if it produces such weird results, why should we accept it as the mathematical notion of "size"? Well, first of all, even if we rejected cardinality as the correct definition of size, we'd still be left with the fact that we can't pair off the natural numbers with the reals, and if that's not because there are more reals than naturals, why is it? Second, it turns out that this concept is much more robust than any other notion of size when applied to infinite collections of things and so weird as it may be, cardinality is the best tool for the job!
Assange
16. August, 2012
Julian Assange is in the news a lot at the moment, and the argument about what should or shouldn't happen regarding him seems bizarrely polarized.
Assange, as most people probably know, runs WikiLeaks which a while ago published a mass of diplomatic cables, generally embarrassing a bunch of bigwigs, especially in the US. The accusation has also been made that the cables potentially put people in danger, but this is rather tricky to ascertain. Not only that, but when WikiLeaks offered to let officials help redact the cables to prevent endangering lives, they were refused.
Separately, Assange has been accused of rape in Sweden. The facts surrounding this are frequently painted in quite moronic monochrome; a supporter will say that all that happened was a condom broke, whilst his detractors will just say he is accused of raping two women. It is clearly wrong to paint the picture as so simple (even should one turn out to be correct) since the charges were bounced between rape and something-bad-but-not-rape (although some people think this is evidence of conspiracy against him) In any case it seems plausible that he did something pretty nasty and ought to be questioned about it, ignoring everything else.
Before he could be brought in for questioning in Sweden, Assange left for the UK. In December 2010, Assange went to a police station in the UK and was subsequently arrested, but later released on bail. He was to be extradited to Sweden for the crimes allegedly committed there. His lawyers appealed, but ultimately the decision was upheld. However in June 2012 he took refuge in the Ecuadorian embassy in London and requested political asylum. This was in breach of his bail conditions, meaning he has now definitely broken the law in the UK, regardless of his guilt in Sweden.
The contention is that the only reason he's being sent to Sweden is so he can be extradited to the United States, who could prosecute him for treason, which carries the death penalty. This would be wrong since Assange has not really done anything to the US apart from publish embarrassing things. Furthermore, several renowned newspapers also published information from the leaks, making them just as culpable for this alleged crime.
But this does not actually warrant the hysteria that surrounds the case all over the web. A trip to the wikipedia page for the European Arrest Warrant (the system used to request that the UK send Assange to Sweden) is enough to inform anyone that Assange cannot be extradited from Sweden to the US without the UK's permission. So sending him to Sweden will make it harder, not easier, for him to be sent to the US.
This, alone puts paid to all the people crying "conspiracy" over the fact that Sweden is refusing to interview Assange in the UK or to guarantee he will not be extradited to Sweden. Even so that is ridiculous; they require Assange to be questioned in Sweden — as, apparently they do of everyone else in this situation — and Assange has no right to demand otherwise. Sweden's judiciary is independent from its government, so their diplomats can no more guarantee they will not extradite him to the US then I can, and asking the courts to decide on a case before it's been given to them is all kinds of stupid. Even without guaranteeing anything though, Swedish law does not allow people to be extradited for crimes carrying the death penalty. You have to be fairly paranoid (or worried they're going to find guilty of sexual assault) to try and use this argument.
Then there is the nonsense with the UK's letter to Ecuador's diplomats. If you believe what Ecuador, the rest of South America, and any of Assange's fans claim about the letter, it contained an explicit threat that the UK was going to bust down the doors guns blazing, snatch Assange and fly away.
What the letter actually did was draw Ecuador's attention to a law "that would allow us to take actions in order to arrest Mr Assange in the current premises of the Embassy." There were of course other more conciliatory things in the letter, and rather less amicable statements that they consider the use of the embassy in this manner incompatible with the Vienna Convention (the bit of international law that governs diplomatic missions)
Of course it's all written in the language of diplomacy, so what looks fairly innocent could rightly be perceived as a threat. But it is facetious to cry about the UK acting imperialist and trampling on international law by storming the embassy, when Ecuador first harboured a criminal (not just an alleged criminal, since by just being there he's breaching bail.)
This is particularly ridiculous since the Vienna Convention expressly prohibits interfering in the host country's domestic affairs (and whether the UK extradites Assange is such) and since what the UK has said it could do is remove the embassy's diplomatic status (should it indeed by found to have violated the Vienna Convention) and then arrest someone who's currently residing there. If this all went according to plan, then the "inviolability" of diplomatic missions which Ecuador's president likes to refer to is entirely irrelevant, since the building won't be an embassy.
It's slightly more complicated than that because embassies have been used somewhat regularly for people to escape political persecution by claiming asylum, and the UK's law seems to say they can intervene whenever this happens. While this is a genuine issue, noone seems to be claiming that it is a requirement of international law that the host of a diplomatic mission allow them to ship alleged criminals out. In any case, it's certainly not being claimed that Assange is facing political persecution in the UK, it's that he might face it if he is extradited from Sweden to the US, which doesn't seem really to be the point of political asylum, and anyway, as mentioned above, will be strictly harder than extraditing him from the UK, since then both the UK and Sweden would have to agree.
On the other hand there are those who cry that he has endangered the lives of soldiers, that he raped two women and deserves everything he gets. This is also premature since there's little evidence that the leaks endangered anyone and, if it did, the people who could really have redacted the cables to prevent that refused to help. And whilst he ought to answer to the Swedish justice system, it's not in the least bit clear what, if anything, he has done that is criminally wrong.
Mmm, maths
8. May, 2012
I went to a conference last week. It wasn't the first conference I've been to, but in some ways it was the first "real" one since the actual first was before I started the PhD, and neither of the ones before this was strictly relevant to my topic.
This was the 5th Young Set Theory Workshop, held at the CIRM, a conference centre in Luminy, near Marseille in the south of France. Since I study set theory, this was definitely a good start.
The surroundings of Marseille are pretty spectacular --- slanted rock strata form impressive, somewhat barren mountains with sparse coverings of scrub. I watched these slide past on the bus from the airport, and again on the bus from the train station, in a slight daze having got up at 4:30 in the morning, with only coach- and plane-sleep to supplement. Nonetheless it was suitably impressive.
Arriving at the conference with old tutor Andrew (whom I'd met at the bus stop, handily settling the question of which side of the road the bus went from) I found old friends David and Greg --- we'd graduated together with PhDs in set theory lined up, so we knew it wasn't "goodbye" and sure enough we'd made it this far.
The days that followed were a pleasant mix of mathematics and socialising. I find it very easy to get on with academics; whether it's that our thinking patterns are similar, their outlooks or something that smacks less of pseudo-science, there seem always to be good humour and a similar curiosity. The latter especially so in such a context when there are many strangers from different cultures.
I was glad that I had learned the rudiments of Forcing, since the majority of talks and lectures touched on the subject in some way. At times I felt pretty behind --- which is to be expected, although it can be disheartening when there are apparently intelligent questions from the audience indicating they have a clue what's going on. However by the end it was clear that, at least among the early PhD students, I was predictably in good company (and in fact several of us opted out of some of the later talks)
Itay Neeman's lecture series was specifically on Proper Forcing --- a technique I hadn't even heard of, but were actually quite understandable. The reason for this is that he actually covered only the basics; this was described as
It was as if, in the first lecture, he showed this beautiful, pristine, vinyl record, just lifting it from the dust jacket. But then he spent the next three lectures building a record player, only playing a tiny snatch of it in the last few minutes and saying "oh, you can come and listen to it at my house!"
So he obviously was left wanting more of the advanced stuff!
Anyways there were also a few talks quite directly related to my work, which is pretty much a first. Likewise it's amazing to be able to talk about my research without simplifying everything; there's a broad spectrum from "lay person" through "general mathematician" to "pure mathematician", "set theorist" and finally "someone who understands what I'm talking about". Even within the conference most people don't understand all the background to a particular person's research.
The social aspect is of course not to be ignored. We all spent long evenings telling jokes and discussing the fine distinctions between British and American English (we call it a "table tennis bat", they call it a "ping pong paddle"!) drinking slightly overpriced French wine. There was also a vending machine that dispensed beer, which was a bit disturbing...
There was a Greek who knew about as much Monty Python as I do, a Canadian who taught us Magic: The Gathering, a Finn who was as serious as the stereotype and who told disturbing jokes and everyone else besides. In the discussion blocks one was free to find someone to talk maths with or chat about this and that. One afternoon we walked out over the hill down to the "calanque" or inlet to paddle in the Mediterranean. (There was also a more intrepid group which hiked up the mountain --- I was not feeling up for this, clearly the right decision in the end as I didn't have nearly enough water and got sunburnt on the shorter walk.) Here I caught up with Greg, who, it turns out, is in a similar situation to me; we both procrastinate too much but have proved one or two rather trivial results that nonetheless serve to boost ones confidence.
On other occasions I told someone who knew about Determinacy what I was doing, and he suggested some interesting things that might be useful in the future. Another time I looked at a generalisation of stationary sets with my supervisor's other student. One other time I told David and Greg how MOBA games like LoL and DotA work.
Before long it was time to return home. For the second time I brought my terrible French to bear to tell the staff that I didn't need lunch on Friday (the first was to check I was boarding the correct train; I was. In fact I was surprised by how much French I remembered, although I certainly forgot almost all of it) and was away at 11AM. 13 hours of travelling later and I was home by 11PM, UK time. Protip: Marseille terminal MP2 is a dive and a sandwich/drink combo costs €7 --- altogether an unpleasant place for your flight to be delayed an hour. Not that being stuck at passport control for nearly an hour at Stansted is much better, especially when you can't even see the passport desks.
Time Passes
29. January, 2012
And life changes. I'm now well into my PhD, which is exciting — I get to do maths all day! It's hard to get up at more-or-less normal times all week, but I'm doing OK at that. For the first year there's a lot of background to get to grips with before you can forge out into new territory. It's harder to motivate oneself to read a book than to do try and prove things, but it's got to be done.
The actual research also gets tantalisingly close, such that it's an effort to ensure one properly learns the material. It's all too easy to jump in before you're ready only to have to retreat again...
Our Maths department at Bristol is impressively sociable; we go to the pub and for a meal each Friday, and go to lunch together every so often as well. Mathematical company can be surprisingly good — it'd be easy to assume that the mathematicians would be as dry as the subject can be, but so far so good.
In November I bought a new computer, which is pretty fun. Unfortunately, it's experienced a whole bunch of problems. This is fairly unusual for me; most of the time computer builds go fairly smoothly. This time one stick of RAM was completely bust, which seemed to result in the OS installation being corrupt, requiring a reinstall. After this I still got new corruption, and after a long time realised that the hard drive was probably dodgy as well. So far a firmware update seems to have fixed the latter problem. Ockham's razor led me astray in assuming everything came from the faulty RAM!
It gets worse though! I sent the RAM back to Scan early in January and it hasn't arrived, so it seems to have been lost in the post. To add insult to injury, my headset broke the other day, which was quite sad; I only dropped it from the desk to the floor. (This is pretty obviously a design fault in the Logitech G35s since numerous other people have had them break in the same way. A very small rod connects the cans to the headband.) Hopefully the third attempt at gluing it will be successful, although I suspect the joint, being quite wide and now composed entirely of glue, will be quite brittle and therefore break before long. I wonder whether a return will be accepted with it covered in glue...
Deutschland
9. September, 2011
I got back from Germany a few days ago after about three weeks in the country. I stayed in Berlin for most of the time, a city I'd never visited before even though I've been to Germany a few times before. I had a few reasons for going; apart from just wanting a bit of a holiday, I'd not done any proper German-speaking for ages and wanted some practice. I've also accumulated a bunch of contacts via the internet over the past years and wanted the opportunity to meet some of them in person.
This was actually my first time going abroad on my own, so it was a fairly new experience in that sense. I'm still a bit mystified that all you have to do is click a few things on the web and then you can travel a thousand kilometres across the sea to another country, culture and language. And then you can stay there for weeks, subsist, talk, make friends, all kinds of things.
I was staying with one particular friend, one of many I'd met in #notpron on QuakeNet — we've been talking for a long time and she'd moved to Berlin from Finland to get a summer internship (another scary and cool thing you can apparently do!) who in turn was sharing a flat with a prolific CouchSurfing host. This meant, in addition to meeting her and the other occupant of the flat, I was guaranteed to meet the many other people who'd be sleeping on the two massive air mattresses in their living room. I'd never done anything with CouchSurfing before, but I'd definitely recommend it — you automatically get to meet fun people from all over the place. We shared languages, sweets, customs (as well as mattresses) with each group.
Berlin itself is a quite incredible city. The German capital seems to have a thing for really wide roads, while still being a big, old city, making it feel quite unlike other capitals I've been to. History is everywhere. All the more present for the fact that so much of it happened within the last 100 years, the reminders of the World Wars and Cold War can be quite sobering. Most of the old buildings bear the scars of WWII; even where the shrapnel and bullet holes have been filled in you can see where the repairs were made. Numerous exhibitions and memorials are dedicated to this period, too.
The Cold War, being even more recent, is even more uncanny. But as someone born in 1988, I was never cognisant of the Berlin Wall when it existed, and only learned about it as an aside in German lessons at 6th form. It was a sort of obvious surprise when the friend I met on my first full day in the city told me that the last time he was in Berlin, he could only see the Brandenburg Gate, not walk through it. The city does a good job of ensuring that it's difficult to forget about the injustices that have been perpetrated within it. The course of the Wall is marked along its length by cobblestones in the ground. In some places it runs through areas of near identical stone, discernible only by the cobbles' contrasting orientations. For one stretch along Bernauer Straße, the former death strip has been filled with an outdoor exhibition containing accounts and information about what happened to the houses that overlooked the border. The contrast between East and West was sometimes so great as to be comical — while the Stasi were trying to shoot you for "defecting", the fire service in the West were out with nets to catch you as you jumped from the windows that hadn't yet been boarded up.
But there was plenty to not be sober about, too. Lots of cities have museums, but Berlin has an entire Museum Island to look around. I didn't visit all the museums on it, but the ones I did visit were suitably impressive (with obligatory snarky comments about the Russians still having some stolen booty from the war) although ultimately the Deutsches Historisches Museum seemed more interesting. Oddly I've not seen a similar attempt in a museum at demonstrating the entire known history of a nation from ancient times to present day in one continuous exhibition. Unfortunately there's a bit too much to get through in one sitting (especially since we visited the special exhibitions first) but even with skimming some parts of it it was very much worth it.
Outside of museums, we did a little urban exploration at Teufelsberg, an abandoned Cold War era radar and listening post, built on an artificial hill formed out of rubble from World War 2. Very rewarding as a first UE site (also easy to get into, and with the rather discordant presence of a bunch of other tourists and young people around eating lunch sitting up a massive radar tower) what with its huge domes which used to surround the listening devices. The day after that we had a pleasant excursion to the almost painfully picturesque town of Potsdam. It's fairly modest in size, but has a massive palace and surrounding grounds, churches, its own Brandenburg Gate and so on. It's almost too nice.
Some high points for me involved meeting a few strangers on two occasions, firstly at a CouchSurfing language exchange meeting and secondly at a party for a friend of a friend. On these occasions I convinced myself that my (nonetheless very much intermediate) skill at German was good enough to be quite fun. Having a couple of conversations without having to search around for words or getting mired in a soup of verbs does great things for self-confidence, even if the only reason you did well was because you've talked about the topic a lot before.
But probably the best thing was being able to finally meet people who up until then were only lines of text on my screen (and occasionally voices over Skype) I'm trying to think as much about them as possible, because meeting someone you've known for nearly 5 years for the first time is quite a strange experience. So strange that one's mind tries to convince one it didn't happen; you must have met them earlier, surely! And living with someone for three-odd weeks, and taking two train journeys each lasting about 8 hours with them, certainly gives one the opportunity to get to know them. A long airport goodbye later and it was back on the way to England where the temperature was 10 or more degrees closer to freezing.
Riots
11. August, 2011
Anyone in the UK not living in a cave will probably have realised that the country has experienced some of the worst disorder for a long time, with riots, vandalism, looting, mugging and crime of all flavours occurring in cities all across England. As the riots were happening and now that they appear to be dying down there has been a lot of debate as to the root cause of the riots and what should be done about them.
First of all it's pretty much clear that the riots have nothing to do with the death at the hands of firearms officers of Mark Duggan. While that was the spark, it's subsequently been made clear that the looters are not concerned about the event.
That in itself doesn't indicate that there is no legitimate grievance amongst the rioters, although this again seems doubtful. We need to be a bit careful when talking about this, though; what I mean is not that those committing crimes have no reason to be angry, nor that there could be no societal responsibility that should have prevented the violence. Rather, that there appears to have been no rational procedure starting with "I'm angry" going through "Because the government/police/etc did something wrong" and, with a bit of stirring, ending up at "Let's riot."
Handily the world has been experiencing protests and riots recently that allow us to make some neat comparisons. If you take, say, the Egyptian protests, it is quite obvious that the people on the streets, and probably even the people torching government buildings, were acutely aware of the problems with the state and why they were left with no choice but to take to the streets. This is in stark and depressing contrast to the pair of girls interviewed by the BBC who were rioting to "show the rich we can do what we want" and "it's the governments fault ... yeah, Conservatives ... or whoever it is, I dunno."
And so, in this sense, I would say, David Cameron is right to describe it as "criminality, pure and simple." It's not really a protest, and it is certainly criminal. Furthermore, there is some sense in which no matter what further analysis may uncover, some of the actions were absolutely beyond excuse or even understanding. To attack the police is, arguably, understandable — if you have been the victim of police oppression all your life, for example. But to attack fire and even ambulance crews as they are attempting to help people is sickeningly, intensely wrong. Nonetheless, as I indicated before, that's not to say that there aren't deeper issues at stake. Something can be "pure criminality" and utterly deplorable whilst still having societal causes that can be corrected by the state.
I was and still am sceptical of many of those commenting and saying that the fault lies with the state. This is because many are claiming that poverty, alienation, disaffection and lack of a stake in society are (correctable) causes of the looting. Yet from reports from witnesses, interviews and video footage we can see that a significant number of those taking part in the looting are unlikely to be poor, alienated and so on. One of those charged in the wee-hours court sessions recently was a University graduate training for her career. Witnesses and footage shows designer gear in great evidence (and not just being carried out under people's arms), and we hear that much of the looting was organised with the help of smartphones.
Now, what has gone unsaid (as far as I'm aware) is that people with smartphones and designer gear cannot be poor, which is probably untrue. But nonetheless I think it is indicative that there is a sizable portion of the crowd who are not disaffected, who have no reason to be lashing out at society and are just there for the thrill and the loot.
But that doesn't show that none of them are, or even that, if we fixed some societal problems, the riots would still have happened. Obviously you need to reach critical mass for an event of this magnitude to spread all across the country, and it seems likely that the well-integrated members of society who took part didn't start the trouble, but got swept up when they saw the opportunity.
Particularly worrying was this video from the Guardian. I was sceptical as to how effective government measures can be to solve problems like those said to be at fault here, but at least in this case it seems the provision of youth clubs is effective and they were not shunned by the people they were open for. Similarly this article includes an insight into how stop-and-search powers really can cause alienation.
For once it seems, though, that the comment is more polarised than parliament. From what I've read of the parliamentary proceedings, there seems to be quite a low (albeit nonzero) level of backbiting and opportunism. (As an aside I'm glad there's little real attention paid to reversing cuts to the police: if your policy is public spending cuts then a single datapoint, even a nasty one, is not enough to legitimately reverse the decision. Our politics already has enough knee-jerk reactions. The mooted restrictions on the use of instant messaging services would be just such a reaction, without even having the benefit of sounding sane)
But most of the comments I've read either condemn the riots and those who seek to find deeper explanations than "the youth of today" as seeking to apologise for the crimes or they condemn the riots and the former bunch of commentators for ignoring the real cause. The truth, I suspect is somewhat inbetween — the rioters are indulging in "pure criminality" yet it's not unreasonable to posit further causes, although whether there is sufficient evidence to make a definite assertion on that subject still seems doubtful.
Perhaps a more pertinent question, though, is "what should be done?" Immediately, of course, we want to quell the violence. After that, though, there is little suggestion of what action can be taken to prevent a repetition. Reopening youth clubs could be a start, although it seems that the fact of deficit reduction is here to stay at least for now, and so this could be difficult to orchestrate.
The role of the police in stopping and searching black men and boys, and no doubt other instances of discrimination are no easier to tackle. Part of this is because when criminal activity is associated with a particular race, discrimination becomes inherently useful. As far as I am aware, black people are overrepresented in street gangs and so if you're going to attempt to use a power like stop-and-search to combat gang crime then of course you're going to target people more likely to be involved. The only sensible alternative is to ditch the power entirely — which may be the correct response, but hardly an easy decision.
Hopefully there are other possibilities that are less difficult to implement, but so far I've not seen people discussing them much. Perhaps we can move on from universally condemning the violence to universally identifying things that can be fixed.
Returning
27. July, 2011
One short holiday removed me from Bristol for a few weeks, but now I'm back and more masterful than ever before! That's right, graduation happened and I'm now a fully-fledged Master in Science! feelsgoodman. In the process I earned an award for the best undergraduate project, which likewise feels pretty good (and is probably some kind of justification/reward for having one's supervisor scare one by saying that the paper might actually be too hard and that it would be no dishonour to go and do something easier) Presumably on account of this the department wants to put me forward for the SET awards (some UK award, basically for projects by undergraduates in science, engineering and technology degrees.) This required writing a synopsis of the project, which ended up being a more drawn-out affair than I expected. I knew it wouldn't be easy to describe the most abstract part of mathematics to a judging panel who are of unknown backgrounds — but presumably not all of whom will know anything about set theory and logic. In the end, though, there was some agonising over how (and indeed, whether) to phrase a sketch argument. Still, the shot at an apparently prestigious award and the accompanying finalists' dinner is surely worth it.
Elsewhere in mathematical goings on I've been graciously awarded money to go to a conference in Chicago (with the authors of the paper which formed the real meat of my project, no less) in September. This is even before term starts for my PhD, but this of little concern. Apparently the workshop will be part mathematical, part philosophical, and at least the philosophical side should be penetrable. It'll be my first time in America, too; a weekend of mathematics, philosophy and a new country can only be fun, I hope.
Before that lies a fuzzy region labelled "GERMANY" on my calendar. At some point I will work out what precisely when I'll be where, but so far it remains uncharacteristically unplanned. It'll be my first time travelling to another country alone, although I have people to meet me on the other side. Some time in the intervening weeks I really ought to exercise my German, for I fear otherwise I'll be left, at least for the first few days, a bit bereft. The trip will be exciting for other reasons though; I'll be meeting in person for the first time a few friends that I've known online for a long time. One in particular for about five years!
Since I've arrived back in Bristol we've had our first house-wide cleaning operation, a success which was suitably followed up with a roast dinner. Hopefully we can prevent a return of the horrors of the previous house by sticking to some kind of cleaning schedule. We've also been attempting to make the most of our spacious new quarters by forcing people to sit in them and be entertained. At any rate, we said we were entertaining them and we're sticking to that story.
Until my departure for foreign lands, though I have to somehow keep myself entertained, a challenge compounded by the summer absence of many University friends. If only their parents provided them with PCs capable of playing games for their stays at home!
Moving House
27. June, 2011
Well university did indeed finish and apparently I get to graduate and do a PhD and so on — hurrah! Unfortunately this was not the end of my travails as we then had to move house.
This was exciting since we've moved somewhere much bigger and for a good price. However all the associated packing, transporting, unpacking and cleaning takes its toll.
Packing took a day or so of lazy work, and in the process blocked my nose and set me sneezing for the next ~48 hours; I actually felt like I had a full blown cold. I guess I am allergic to dust or something in it (although I will claim I am allergic to cleaning or work or something) which made the whole process a lot more annoying than it had to be.
Next we had to transport all the stuff over to the new place. This was completed in stages, firstly some car-loads with one housemate's vehicle — just enough to be able to sleep there and not starve. At this point I realised that it was unavoidable that I would at some point end up either at the old or new house with something that I needed only being at the other. This realisation was annoying, but not as annoying as when I ended up in the opposite house to my toothbrush.
The next stage was to transport a massive load of stuff with the help of another housemate's Dad and a hired Transit van. We loaded the thing up fully twice, with the second load being part full of rubbish. Even then we ended up with a fair amount of stuff left; this housemate has a lot of stuff!
By this point we were pretty much moved in; timed perfectly to coincide with the installation of The Internet. A trip back to sell the old washing machine to some miscellaneous people allowed another armful of stuff to be moved over, and before we knew it, the Apocalypse had arrived. That is, it was time to clean the old house.
First a little context: Our house was (and our new flat is) made up of four young men. Our attitudes to cleaning vary from apathy through hate to mild enthusiasm. The sum of this was definitely not enough to complete serious cleaning very often in our small, somewhat grotty student house. Thus a full weekend was allocated for cleaning, and over we went.
The Dust attacked again, but this was nothing compared to the horrors awaiting us in the two bathrooms and one kitchen that the house had yet to throw at us. One person had the task of the main bathroom. Apparent acres of tiles, grouting and sealant awaited him, awash in a bilious sea of black mould. Of course, the black mould doesn't come out, so that just makes the job disheartening rather than difficult. Having not had a really thorough clean for about five years, the walls had seen the swipes of thousands of greasy fingers, which had to be cut through armed only with a jay cloth and a spray bottle.
The worst denizen, though, was in the kitchen. Naturally a kitchen acquires its expected layer of grease-mist over everything. For this, we were prepared. We were, too, prepared for the oven being fairly horrible, but not to the level required to face...
The Substance.
The Substance, as we have dubbed it, must be what happens to... things you cook when you subject them to roughly 180 degrees followed by cooling to room temperature repeatedly. However, it does not share its properties with any food substance or component that we cared to consider.
It is black, smooth and shiny. It is a flexible solid that can be torn with the fingers. It has the consistency of jelly but is somewhat tougher, and will snap into pieces if bent too far. It is clearly neither fat, nor carbon, nor sugar. It could be gelatine, but the tenacity with which it clings to the oven (the best tools we have for extracting it are steel wool scourers and knives) does not support this hypothesis.
Whatever it is, it is fairly disgusting, is not penetrated by regular oven cleaners and takes ages to shift.
Anyway once all that is done I'm roughly free. Still got to finalise the bills for the new place (I am either terrible at life or there is no sensible way to transfer providers at the same time as moving) and reclaim some tax (which requires me to complete a paper form as do some other financial things — Inland Revenue needs to move with the times!) and go on holiday twice, graduating in between.
Who thought finishing work could be so much... work!
Endings
26. May, 2011
So the University year is drawing to close, and I have only one thing left to do. It's quite weird to think that an entire chapter of my life is ending, but sitting right in the middle of it, nothing really seems to be changing, not yet anyway.
When I was younger I used to wonder whether, when I was older, there would be any points where my life would change drastically, and tried to imagine possible times. Starting my first full-time job was the only one I can remember; the recurring problem was that I would get to the point I'd imagined and be unable to recall what I had imagined it would be like, and so not be able to compare. I'm not even sure what I imagined starting work would be like, but (if you call a PhD work...) I can't imagine it will be that much of a jarring transition. And if you don't call a PhD work, then it will be even less of a change to go from there to post-doctoral work or whatever.
Anyway, we shall see whether I do just float gently through the waters of academia, starting in a few months.
In the news recently has been a somewhat mystifying frenzy over Ken Clarke's comments regarding rape. I can't decide whether I am actually in the minority of opinion on this topic, or whether the media is just overburdening the poor horse drawing this bandwagon. Certainly, Ken dropped a few clangers, but to suggest that admitting degrees of seriousness for serious crime makes the crime seem less serious is fairly bizarre. The example Clarke gave was a good one although apparently it's not necessarily rape under UK law, but nonetheless it's not too hard to dream up an example of rape that is, while still well on the serious side of the scale of serious to non-serious, is nonetheless lower than some other example.
Before I continue, perhaps it would be prudent for me to stress that I consider rape a serious crime for which the responsibility always lies with the offender. (although statutory rape, if it turns out to exist, perhaps does not fall into this category. In the eyes of the law, no consent would have been given, but in reality it's a little more complex at least) Further I should probably just apologise in advance in case anyone reading this gets offended or angry. Nonetheless, this is what I think and I'm going to write it down.
Most things I've read have people arguing that admitting this amounts to saying that rape is not serious, which is such a blatant non-truth it's difficult to understand why anyone would say it. No-one supposes that because murder is pretty bad, you can't have even worse murder.
A more interesting contention is that allowing degrees of seriousness, while not actually implying that the umbrella category is overall less serious, might cause people to nonetheless thing that way. This is more of a possibility, but in practical terms I think it is pretty much never worth trying to second guess the public in this way. If, it turns out, it is worth making the distinction — and by all accounts though, it seems the case that a judge will hand out more or less severe penalties to what are being described as more or "less" serious rapes — then it should be done, and it is up to the government or some public body to ensure that this does not do anything to increase crime, decrease the reporting or conviction of crime, and so on. (Perhaps it would be worth getting Orwellian to satisfy people on this subject - we could have serious and doubleserious rapes... hopefully this will be taken as a statement about the camp of dissatisfied people, not about rape.)
At the risk of further enraging people with controversial opinions about a sensitive topic, I find it similarly odd that people are so loath to allow even a proportion of blame with victims, as if we live in a world where blame is assigned to one and only one person for every event. This came to the fore recently with the police officer who told a bunch of students not to dress sluttily (perhaps not his precise words) in order to help prevent rape. Now, it's pretty much a given that you can't make such statements without being explicit about what you're not saying. For example, if you're going to say that, you should make it clear you're not saying that rape is "the fault" of the victim.
But it is still a simple question of fact as to whether wearing revealing clothing increases ones chance of being raped, and if it does then it is arguably prudent to avoid it. Similar but less controversial advice is to avoid getting so drunk that you pass out, and to guard your drink. If one doesn't follow this advice, the chance of becoming the victim of rape is increased and so, in some sense, the victim acquires some measure of responsibility. The fact that the question is never "how much" but rather "whether" is confusing, because if you knowingly act so as to increase the probability of something happening, it seems to follow immediately you take on some proportional amount of responsibility. The fact that the ultimate responsibility can lie with someone else is not in question; only the balance of proportion is.
Of course, in the particular case of the police officer, I don't think the somewhat philosophical question above even enters into it. Advice is advice and should be judged on its practicality, not on its perceived implications. It would not be advisable for a white person (to purposefully choose an uncommon example) to walk through some parts of Zimbabwe, or into some bars in India, where whites are generally seen as signs of colonial oppression and might suffer violence simply for being there. The response we see here would be like rejecting this advice as being racist; it is not racist, it is acknowledging that racists exist and are willing to hurt people, and if you want to stay out of harms way you can and should take steps to avoid it. Acknowledging that rapes happen and advising on methods of avoiding it can coexist with other strategies for preventing rape (for example, locking up rapists) and does not in any way reduce the responsibility of the offender.
Alternative Vote
2. May, 2011
So, we're having a referendum on the voting system on Thursday, which is pretty cool. What hasn't been cool is the campaigning that's been done — more so from the No campaign, but true also of the Yes people. Before I go further I should state my allegiances, which lie with AV. In fact I would prefer a system of proportional representation, but I think that is true of a lot of people who will be voting Yes.
My main issue with the arguments being levelled is that the majority of them, either by number or by weight accorded, are missing the main point. In my opinion, voting reform is fundamentally about what is fair, not about who benefits. Of course, no-one is going to act without regard for the practical consequences likely to follow, but the voting system, as we know, is not something that is changed easily, and to vote either way out of concern for short term gains or to "punish" someone is short-sighted and somewhat disgusting.
There will always be problems in disentangling the arguments because the people saying their favoured system is fair are generally the same ones whose party stands to gain from it. That said, I've not heard any arguments tackle head on in just what way FPTP is fairer than AV when faced with just how prevalent "tactical voting" seems to be. Which is perhaps not surprising; to do so would require someone to honestly declare they think it is fairer to vote categorically and unambiguously for someone other than the one you want to win than to be able to list a few preferences in order.
So far I've had two pieces of No literature through the door, and it is true that the second is better than the first. That said I think this is mainly because it's shorter, but no longer are they claiming that some people get more votes under AV (presumably because their votes get transferred, but this seems to be quite a fundamental misunderstanding of what it means to be transferred.) Nor are they claiming it is too complicated. I like to think the No campaign realised they were making themselves look too dim-witted to understand the system (which really isn't complicated, although of course more complicated than FPTP) but really — all the voter needs to understand is that they list any candidates they wouldn't mind winning in order of preference. If they want to know what happens after that, it is not hard to find out and, after all, the number of countries which use STV or D'Hondt is high, and those are pretty crazy in comparison. AV is sufficiently easy that organisations everywhere (my own favourite, COGS, uses a near-equivalent method with runoffs in the case of no absolute majority) use it at their AGMs and so forth to elect just about anything.
They're still saying that AV will lead to more hung parliaments (probably true) but neglect to mention that plenty of countries manage with coalition governments, that no-one will vote for the Lib Dems next election anyway and that the people in favour of keeping FPTP by-and-large approved of the "broken promise" we're all thinking about anyway; talk about preaching to the choir. Of course, it'd be more likely that we'd have Labour and Lib Dem coalitions, and so they'd be more likely to more or less agree on more policies, which again would mitigate this allegation.
They're also still spreading the downright lie that AV will cost £250m to implement. By now anyone who has been following the debate knows that this figure includes £130m on installing imaginary electronic vote counters (other legislatures do not use these to count votes for AV, and there is no intention of installing them in Britain). This is the first lie. The second is that it includes the cost of the referendum itself, which is, needless to say, already spent and not a reason to vote No. This has understandably riled the Yes campaign enough that they have even muttered about legal action. Perhaps futile, but this is electoral fraud of the grandest scale, and I really hope it does not disappear into the mire of old news after the referendum.
On the other side of the debate, the main irritation I have with the Yes campaign is their cheap stab with the expenses scandal. I am fairly convinced that fiddling expenses is a fairly low priority in the grand scheme of British politics, and if there is a culture of doing so the entire blame cannot be lumped solely on the individuals. The ensuing media frenzy still seems to have people convinced that people who tweak their expenses are the root of all that is wrong with the world, but I suspect that the ethos that it was "alright" to charge for flatscreen TVs could easily have survived whatever the voting system. It is worth noticing also, that the MP they like to cite — the one who charged for polishing his moat or something — had the support of over 50% of his constituency.
"Greater accountability" is a nice woolly term that is bandied about by the Yes party too. While it might be true that MPs will be worried about maintaining a broad base of support while in parliament, I doubt that you will change such an unquantifiable thing quickly or with any one measure. Regardless, this must come secondary to whether or not the right people end up in parliament.
But as I stated initially, my core anger is that so many people seem to be advocating voting no to "give Clegg a kicking" or to "vote Yes to keep the Tories out." For the first, Clegg is going to have more of a kicking than anyone could ever administer in a referendum at the next general election, and indeed at the coming local elections. The second I can more identify with, of course, but on its own it's way wide of the point, which is that the Tories should (in general) not be in government because that's what most people would prefer, i.e. that's what they'd democratically choose.
The same goes when talking about the BNP (who come up whenever the voting system is mentioned.) Now, quite apart from the fact that it would be impossible for the BNP to gain the support of 50% of a constituency (which, I feel like adding at this inopportune moment, is not necessary if people don't list enough preferences, although I guess near enough correct for a headline) I find it useful to point out that the BNP got more votes than the Greens yet the Greens have a seat in parliament. There really is no possible way that this can be considered "fair" and, ultimately and regrettably, if a bunch of people vote for the BNP it is their right that they be represented fairly. Now even in fully proportional systems there are usually measures to ensure that parliament doesn't get swamped with a ton of small, extreme parties, and this would likely exclude the likes of the BNP, but this is not inconsistent with them being fair, because a bunch of small parties is undesirable for other reasons. But to vote on the voting system with a specific party's gains or losses in mind as a significant point is overly cynical.
The real shame is that there is a real debate to be had. If you head over to YouTube there are people there hashing out the pros and cons of FPTP and AV, whether tactical voting is truly eliminated (it's not, but it's made so difficult and unreliable as to be pointless given the political landscape in Britain) and getting on with inserting kittens into everything. But this important discussion has been rejected in favour of mudslinging. Hardly surprising, but deeply disappointing, especially if, as it appears will happen, the No campaign wins out. (Although, from what I've heard, the campaigning might not have had much effect either way)
For my part, I'll be voting Yes on Thursday because I think AV is a fairer system than what we have now, because it makes tactical voting difficult enough that people will probably stop doing it, resulting in a parliament more representative of the people's preferences. Analogies to races and appeals to "one man, one vote" are presupposing that the system ought to work like a race, where the person judged to be better than any other individual candidate is the best. This is as opposed to an election, where the person who gains the most support from the electorate should win, and since people can rationally support more than one candidate in differing amounts, this should be reflected in who is elected. Some real information on the relative benefits and shortcomings of AV can be read on this wikipedia article, and see also the main article on AV.
Portal 2
29. April, 2011
Exams are finished and the 4th year project is near complete so I thought I'd say something about That Game. Some small spoilers follow.
It was good, but not as good as I was hoping, which is probably what I should have expected. Part of the problem with reviewing (or "talking about", since this is hardly a review) Portal 2 is that there are two very different halves to it - the puzzles and the humour. I think I'd tentatively say that, in both games, the humour is more important and the thing that makes them stand out.
However, humour is largely a matter of taste. Not that that will stop me explaining how and why I did not find Portal 2 as funny as I remember Portal being. Wheatley was quite hilarious, although this seemed to tail off after the swap. The moment that stood out as the funniest though was right at the start with the "can you talk?" after you wake up - which is a shame, because that is a distant memory by the end of the game.
The end of the calm, dystonic evil GLaDOS was unfortunate, I felt. She's not as funny when being emotional - either evilly so or otherwise. In a way the coop reminded me of the old GLaDOS and I think I found her much funnier there. At least she's not Cave Johnson though, who, while definitely amusing in his first few appearances, took a sharp dive after that you realise he's as one dimensional as the real line (or maybe less). A redeeming feature is that he is the spitting image of Alexandr Buinov of youtube fame.
With the humour out of the way though, I must say I was distinctly unimpressed with the puzzles. The original was not exactly a game on which you'd get stuck for hours, but it hardly seems too cynical to wonder if they intentionally made this one easier for the console crowd. This Nerf NOW! comic pretty much sums up one massive problem. In the original, as far as I remember, you were generally faced with a white (read: portlable) environment with a few surfaces on which you couldn't place portals where that was important for the puzzle. This varied as you went through, but in general working out where to put portals was a big part of the challenge.
In Portal 2 you almost always know where one portal goes: it's underneath a gel dispenser or opposite a funnel or something like this. The other end might be up to you, but it's often the case that you just have to pick between one of a few white squares (which even snap your portal so it lines up nicely) This vastly simplifies the puzzles, and turns it in many cases of a brute-force "imagine each portlable surface in combination with every other one" game. I like puzzles, especially logic puzzles, and there are some brilliant ones you can do with portals and all the new stuff they added, but I don't feel like there are any.
This also led to many situations where I didn't know where I was going next, but could tell what to do simply because of the available surfaces. "Oh, there's a slanted surface, I'll fling myself out of there and see where I end up" went one example. In another, there were two squares on a wall in front of a stretch of solid walkway. Since there was a timed element on this puzzle, it was an immediate conclusion (we didn't have to even try out the level to determine it) that speed gel needed to be placed on the floor.
Of course, just making things harder doesn't always make them more fun. But in terms of difficulty, Portal 2 feels like half a game. You learn to use each of the elements - portals, flinging, cubes, turrets, lasers, bridges, gels and funnels, but then the game is finished. You hardly have to do anything except work out what each one does, leaving me unsatiated. Not to mention that, with all these new puzzle elements, we are hardly challenged to fit them together. There are a couple of levels involving putting gel in the funnels and shielding or stopping yourself with the walls while in funnels, and putting gel on bridges, but these are maybe one example of each combination, and there could be so much more. Even at the expense of dropping a puzzle element entirely I think it would be better to combine them more, because it feels like some were just thrown in for the heck of it.
With puzzle games, I would not expect to be able to finish one in a single sitting without the aid of a walkthrough. Towards the end of the game, at least, there should be some puzzles that take a bit of thinking to crack, otherwise they hardly deserve to be called puzzles. The co-op did get us stuck a couple of times, but only for a minute or so. That said, when you have two minds attacking a project you are less often stuck looking at a problem the wrong way, so this is not a great comparison to single player puzzle games. The fact remains though that only when I was dead tired did I ever stop playing due to not seeing a solution. Upon returning, the answer was pretty much obvious.
And to return to the end of the game and matters purely of taste, I felt the credits song was a flop. Of course it would never have the same element of surprise as the first one, but nonetheless — Still Alive made me grin the whole way through, this one made me smirk a couple of times.
Now, I remember saying at the start of the post that I thought Portal 2 was good, so I feel I ought to apologise for what amounted to a rant, having essentially only focused on the bad aspects. The puzzles were fun and it was funny, but — especially after so long — I hoped for more.
And on one final and terrible note, Valve had the temerity to get Russell's Paradox wrong, which I consider nigh-on unforgivable. There's no inherent contradiction with "The set of all sets," (although normal set theory does derive a contradiction in combination with other axioms) unlike with "The set of all sets which don't contain themselves." Sad mathematician was sad.
Exams, Portal 2
19. April, 2011
Today, three forces that had been building for weeks culminated in a massive implosion of procrastic energy. The first element: Exams. A necessary component, as they create the environment in which procrastination begs to be undertaken. The second: the completion (or near completion) of this website. The third: the release of Valve's Portal 2.
And so it is that, for the past three weeks or so, rather than revising solidly each day I have been working for approximately 5-minute snatches, interspersed with hacking away to python code and CSS. In retrospect it was perhaps not the best of ideas to install Django (the framework this site is built with) mere weeks before the final examinations of my undergraduate career. In retro-retrospect, it was almost certainly a bad idea to buy a VPS to host the website on a few weeks before that, but we live with the follies of the past.
It's true, though, that website production is an excellent distraction for the bored student since it provides a perfect juxtaposition with what you're supposed to be doing. That is to say, it is interesting, intellectually stimulating and gives you a sense of satisfaction when you complete some of it. I can't help but assume that my "revision technique" as some people call it is pretty abysmal — write out key points of course on a sheet or two of A4, do all the exercise sheets (usually finishing most questions with "etc" or "... blabla" after getting bored) and finally do the past exam papers. The last point is a key one since it lets you know just how much detail is expected of you, as well as the flavours of question likely to be asked. Apart from that though, and maybe a little bit of staring blankly at the course notes as a PDF (or my handwritten ones if the former are bad or non-existent) not much happens apart from procrastination.
This has so far not been a hindrance but I'm still left wondering, with one exam left to go (in my entire undergraduate life, perhaps forever) if it's about to come and bite me. Certainly others seem to have more proactive or focused methods, involving flash-cards and... doing things more than once. And libraries. With a solid day left for the final one though, these thoughts are only ever going to be just that. I seemed to recall promising myself a few weeks ago that I would work harder and more solidly than I had the previous year, but obviously that wasn't going to happen.
Portal 2 has been less of a practical consideration in terms of procrastination, since it was released in the wee hours this morning and, although there was a small voice trying to convince me of the opposite, I was never really going to stay up until 5:30 in the morning (let alone get up at 5:30 in the morning) to play it, so I satisfied myself with trying out the first 10 minutes after I woke up, before getting down to the hard slog of avoiding last-minute revision for the exam this afternoon.
If you're not hanging off Valve's every release, Portal 2 was the subject of an Alternate Reality Game that consumed the time of geeks the world over (although even I recognised that obsessing over obscure puzzles and challenges for hours on end would likely be unhealthy, physically and academically.) The culmination of this hype, that started months ago with the trailers, was that if you played (or, it turned out, had running) various indie games you could accelerate the release of the game. This was all a little tired, in a very real sense, in the UK, when it transpired that the efforts going towards getting the game released early only made the difference of a few hours, and put it at a time when we're all in bed anyway. (If you're not a filthy student or something then you probably still have to wait until getting back from work, too.) Nonetheless it was fun to see a new kind of hype surrounding a launch.
Anyway, it's time to sleep to engage in a final day of exam-related non-work tomorrow. On Friday, I can commence engaging in coursework-related non-work.
Welcome!
19. April, 2011
Hello, and welcome to the new fishface.org.uk (Not that there was an old one.) Now that I've (almost) finished the website it's time to put some (garsp) content on it.
If, for some reason, you're interested, the website is powered by Django and nginx. I plugged most of the website together from Django's pool of reusable apps, but there's a significant amount of gluing together which kept my busy for a good while. The best thing about writing your own website (more-or-less) is inline More on that story later.